Source: PYPL Popularity of Programming Language, Feb 2024.
- The PYPL Popularity of Programming Language Index is created by analyzing how often language tutorials are searched on Google.
- Worldwide, Python is the most popular language, Python grew the most in the last 5 years (2.6%) and Java lost the most (-5.3%). Read more here.
- Python is one of the most important programming languages to learn when becoming a data scientist. However, to truly master Python, learning by doing is essential. This is where Python projects come in.
- In this post, we will walk through tested and implemented 100 hands-on Python projects and source codes with useful resources for beginners and beyond.
- These 100 mini projects with solutions are essential for applying learned concepts and understanding how to put them into practice.
- This guide will help you build confidence in the Python learning journey, develop a specific application that helps you stand out in the job hunt, and have fun along the way.
- The post concludes by encouraging readers to check out the resources with project links for inspiration and finding project ideas.
- Explore the world of Python programming with these highly engaging user-friendly projects!
Table of Contents
- Setting Up Your Environment
- Download Datasets
- Initial Pandas Data QC
- Displaying Pandas Data Types
- Showing Descriptive Statistics
- Exploring the Dataset
- Email Slicer
- User Input & Type Conversion
- Working with Lists
- Practicing Loops
- Calculator
- Temperature Conversion
- ADC Temperature Sensor
- Sorting Numpy Arrays
- Story Generator
- Display Calendar
- Invoice Generator
- Using Strings
- Guess a Word
- Tip Calculator
- Pizza Deliveries
- Highest Score
- Password Generator
- Paint Area Calculator
- Menu-Driven Program
- Datetime Module
- Counting Digits
- Largest Number
- Join Two Strings
- Format Floating Point in the String
- Raise a Number to a Power
- Working with Boolean Types
- If Else Statement
- Using AND/OR Operators
- Switch Case Statement
- While Loop
- Use of regex
- Use of getpass
- Use of Date Format
- Add/Remove the Item from a List
- Slice Data
- Add and Search Data in the Set
- Count Items in the List
- Define and Call a Function
- Using Try-Except Blocks
- Read/Write Files
- List Files in a Directory
- Read/Write w/ pickle
- Use of range Function
- Use of map Function
- Use of filter Function
- Pandas First Program
- Current Weather
- Turtle Race Game
- Must Watch Movie List
- Digital Clock
- BMI Calculator
- YouTube Downloader
- Factorial of a Number
- Numpy Linear Algebra
- Numpy/Matplotlib Images
- Numpy Financial Module
- Creating Pandas Data Objects
- I/O Pandas DataFrames
- Tkinter Sentiment Detector GUI
- Tkinter/Pillow Slideshow
- IceCream Debugger
- Using Pandas DataFrames
- Tkinter Calendar
- Loan Calculator GUI
- Weight Converter GUI
- Age Calculator GUI
- Create Dictionary from an Object
- Check a Key in a Dictionary
- Add a Key-Value Pair to the Dictionary
- Iterate Over Dictionaries Using for Loop
- Check the File Size
- Working with Functions
- Working with Dictionaries
- Remove First N Characters from a String
- Working with Classes
- Define Class and Method
- List Operations via Classes
- Minimize Lateness
- Compute a Polynomial Equation
- Creating Linked Lists
- CockTail Sort Algorithm
- Binary Search via Recursion
- Find Simple Interest
- Probability Distributions in SciPy
- Piecewise Linear 1-D Interpolation in Numpy
- Cubic Spline 1-D Interpolation in Scipy
- Interpolation with Radial Basis Function
- SciPy T-Test
- KS-Test
- Statistical Description of Data
- Exporting Data in Matlab Format
- Import Data from Matlab Format
- SciPy Optimizers
- Working with Spatial Data
- Python Coding Interview Q&A
- Conclusions
- The Road Ahead
- Explore More
- References
Setting Up Your Environment
- Setting working directory YOURPATH
import os
os.chdir('YOURPATH') # Set working directory
os. getcwd()
python3 -m pip install requests pandas matplotlib
- Within the Jupyter notebook, this command looks as follows
!pip install requests pandas matplotlib
- You can also use the Conda package manager
conda install requests pandas matplotlib
- Since we’re using the Anaconda distribution, then you’re good to go! Anaconda already comes with pandas and Jupyter notebook installed.
Download Datasets
- Let’s analyze NBA results provided by FiveThirtyEight in a 17MB CSV file. Create a script
download_nba_all_elo.py
to download the data:
import requests
download_url = "https://raw.githubusercontent.com/fivethirtyeight/data/master/nba-elo/nbaallelo.csv"
target_csv_path = "nba_all_elo.csv"
response = requests.get(download_url)
response.raise_for_status() # Check that the request was successful
with open(target_csv_path, "wb") as f:
f.write(response.content)
print("Download ready.")
Output:
Download ready.
- When you execute the script, it will save the file
nba_all_elo.csv
in your current working directory YOURPATH.
Initial Pandas Data QC
- Now you can use pandas to take a look at your data
import pandas as pd
nba = pd.read_csv("nba_all_elo.csv")
type(nba)
pandas.core.frame.DataFrame
len(nba)
126314
nba.shape
(126314, 23)
nba.head()
gameorder game_id lg_id _iscopy year_id date_game seasongame is_playoffs team_id fran_id ... win_equiv opp_id opp_fran opp_pts opp_elo_i opp_elo_n game_location game_result forecast notes
0 1 194611010TRH NBA 0 1947 11/1/1946 1 0 TRH Huskies ... 40.294830 NYK Knicks 68 1300.0000 1306.7233 H L 0.640065 NaN
1 1 194611010TRH NBA 1 1947 11/1/1946 1 0 NYK Knicks ... 41.705170 TRH Huskies 66 1300.0000 1293.2767 A W 0.359935 NaN
2 2 194611020CHS NBA 0 1947 11/2/1946 1 0 CHS Stags ... 42.012257 NYK Knicks 47 1306.7233 1297.0712 H W 0.631101 NaN
3 2 194611020CHS NBA 1 1947 11/2/1946 2 0 NYK Knicks ... 40.692783 CHS Stags 63 1300.0000 1309.6521 A L 0.368899 NaN
4 3 194611020DTF NBA 0 1947 11/2/1946 1 0 DTF Falcons ... 38.864048 WSC Capitols 50 1300.0000 1320.3811 H L 0.640065 NaN
- Let’s customize configuration settings
pd.set_option("display.max.columns", None)
pd.set_option("display.precision", 2)
nba.tail()
gameorder game_id lg_id _iscopy year_id date_game seasongame is_playoffs team_id fran_id pts elo_i elo_n win_equiv opp_id opp_fran opp_pts opp_elo_i opp_elo_n game_location game_result forecast notes
126309 63155 201506110CLE NBA 0 2015 6/11/2015 100 1 CLE Cavaliers 82 1723.41 1704.39 60.31 GSW Warriors 103 1790.96 1809.98 H L 0.55 NaN
126310 63156 201506140GSW NBA 0 2015 6/14/2015 102 1 GSW Warriors 104 1809.98 1813.63 68.01 CLE Cavaliers 91 1704.39 1700.74 H W 0.77 NaN
126311 63156 201506140GSW NBA 1 2015 6/14/2015 101 1 CLE Cavaliers 91 1704.39 1700.74 60.01 GSW Warriors 104 1809.98 1813.63 A L 0.23 NaN
126312 63157 201506170CLE NBA 0 2015 6/16/2015 102 1 CLE Cavaliers 97 1700.74 1692.09 59.29 GSW Warriors 105 1813.63 1822.29 H L 0.48 NaN
126313 63157 201506170CLE NBA 1 2015 6/16/2015 103 1 GSW Warriors 105 1813.63 1822.29 68.52 CLE Cavaliers 97 1700.74 1692.09 A W 0.52 NaN
Displaying Pandas Data Types
- You can display all columns and their data types with
.info()
:
nba.info()
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 126314 entries, 0 to 126313
Data columns (total 23 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 gameorder 126314 non-null int64
1 game_id 126314 non-null object
2 lg_id 126314 non-null object
3 _iscopy 126314 non-null int64
4 year_id 126314 non-null int64
5 date_game 126314 non-null object
6 seasongame 126314 non-null int64
7 is_playoffs 126314 non-null int64
8 team_id 126314 non-null object
9 fran_id 126314 non-null object
10 pts 126314 non-null int64
11 elo_i 126314 non-null float64
12 elo_n 126314 non-null float64
13 win_equiv 126314 non-null float64
14 opp_id 126314 non-null object
15 opp_fran 126314 non-null object
16 opp_pts 126314 non-null int64
17 opp_elo_i 126314 non-null float64
18 opp_elo_n 126314 non-null float64
19 game_location 126314 non-null object
20 game_result 126314 non-null object
21 forecast 126314 non-null float64
22 notes 5424 non-null object
dtypes: float64(6), int64(7), object(10)
memory usage: 22.2+ MB
Showing Descriptive Statistics
- To get an overview of the values each column contains, you can use
.describe()
:
nba.describe().T
- Output:

Exploring the Dataset
- Exploratory Data Analysis (EDA) can help you answer questions about your dataset
#Examine how often specific values occur in a column
nba["team_id"].value_counts()
BOS 5997
NYK 5769
LAL 5078
DET 4985
PHI 4533
...
INJ 60
PIT 60
DTF 60
TRH 60
SDS 11
Name: team_id, Length: 104, dtype: int64
- It seems that a team named
"Lakers"
played 6024 games, but only 5078 of those were played by the Los Angeles Lakers. Find out who the other"Lakers"
team is:
nba.loc[nba["fran_id"] == "Lakers", "team_id"].value_counts()
LAL 5078
MNL 946
Name: team_id, dtype: int64
- Then you can use the
min
andmax
aggregate functions, to find the first and last games of Minneapolis Lakers:
nba["date_played"] = pd.to_datetime(nba["date_game"])
nba.loc[nba["team_id"] == "MNL", "date_played"].min()
nba.loc[nba['team_id'] == 'MNL', 'date_played'].max()
nba.loc[nba["team_id"] == "MNL", "date_played"].agg(("min", "max"))
Output:
min 1948-11-04
max 1960-03-26
Name: date_played, dtype: datetime64[ns]
Email Slicer
- Trying the Email Slicer that will take an email address as input and slice it to produce the username and the domain associated with it.
email = input("Enter Your Email: ").strip()
username = email[:email.index('@')]
domain = email[email.index('@') + 1:]
print(f"Your username is {username} & domain is {domain}")
- Example I/O
Enter Your Email: metava922@gmail.com
Your username is metava922 & domain is gmail.com
User Input & Type Conversion
name=input("what is your name? ")
print("Hello {} !".format(name))
print(type(name))
num1=input("write a number")
num1=int(num1)
num=5
type(num)
result=num+num1
print("{}+{}={}".format(num,num1,result))
- Example I/O
what is your name? Al
Hello Al !
<class 'str'>
write a number15
5+15=20
Working with Lists
- Learning about lists with the help of the following example
a=[14,15,"Said"]
b=[14,58,56]
b_copy=b[:]
c=a+b
print(c)
print("a:{} and b:{}".format(a,b))
print("a's location is {}, b's location is {}.".format(id(a),id(b)))
a[0]=165
print("a's location is {}, b's location is {}.".format(id(a),id(b)))
print(b_copy)
family=["Said","Maureen","Mina","Fatima"]
print("first letter of each one: {},{},{},{}".format(family[0][0],family[1][0],family[2][0],family[3][0]))
- Example I/O
[14, 15, 'Said', 14, 58, 56]
a:[14, 15, 'Said'] and b:[14, 58, 56]
a's location is 2582634989888, b's location is 2582634988736.
a's location is 2582634989888, b's location is 2582634988736.
[14, 58, 56]
first letter of each one: S,M,M,F
Practicing Loops
- Let’s see how loops are used to iterate over sequences such as lists, strings, tuples, etc.
#While_loop
names=["Bob","Jack","Bob","Alpha","Astra","Bob"]
n=names.count("Bob")
while n>1:
names.remove("Bob")
n-=1
print(names)
['Jack', 'Alpha', 'Astra', 'Bob']
- for Loop example
languages = ['Swift', 'Python', 'Go']
# access elements of the list one by one
for i in languages:
print(i)
Swift
Python
Go
Calculator
# This function adds two numbers
def add(x, y):
return x + y
# This function subtracts two numbers
def subtract(x, y):
return x - y
# This function multiplies two numbers
def multiply(x, y):
return x * y
# This function divides two numbers
def divide(x, y):
return x / y
print("Select operation.")
print("1.Add")
print("2.Subtract")
print("3.Multiply")
print("4.Divide")
while True:
# take input from the user
choice = input("Enter choice(1/2/3/4): ")
# check if choice is one of the four options
if choice in ('1', '2', '3', '4'):
try:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
except ValueError:
print("Invalid input. Please enter a number.")
continue
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
# check if user wants another calculation
# break the while loop if answer is no
next_calculation = input("Let's do next calculation? (yes/no): ")
if next_calculation == "no":
break
else:
print("Invalid Input")
- Example I/O
Select operation.
1.Add
2.Subtract
3.Multiply
4.Divide
Enter choice(1/2/3/4): 1
Enter first number: 45
Enter second number: 22
45.0 + 22.0 = 67.0
Let's do next calculation? (yes/no): no
Temperature Conversion
- Convert Fahrenheit to Celsius or vice versa.
- Convert Celsius To Fahrenheit
celsius = float(input("Enter temperature in celsius: "))
fahrenheit = (celsius * 9/5) + 32
print('%.2f Celsius is: %0.2f Fahrenheit' %(celsius, fahrenheit))
- Example I/O
Enter temperature in celsius: 23
23.00 Celsius is: 73.40 Fahrenheit
- Convert Fahrenheit to Celsius
fahrenheit = float(input("Enter temperature in fahrenheit: "))
celsius = (fahrenheit - 32) * 5/9
print('%.2f Fahrenheit is: %0.2f Celsius' %(fahrenheit, celsius))
- Example I/O
Enter temperature in fahrenheit: 100
100.00 Fahrenheit is: 37.78 Celsius
ADC Temperature Sensor
- Temperature sensors in real life read a voltage value that represents the current temperature, then this value goes through an ADC (Analog to Digital Converter), which converts the analog signal to a digital one resulting in what we call ADC raw value.
- Creating a virtual temperature sensor
SYSVOLT = 5
ADC_RESOLUTION = 4095
MAX_VOLT = 2.442
MIN_VOLT = 0
MIN_TEMP = -50
MAX_TEMP = 50
def adc_raw_value(v):
if(v >= MIN_VOLT and v <= MAX_VOLT):
ADC = (v*(ADC_RESOLUTION/SYSVOLT))
return round(ADC)
else:
return None
def adc_to_c(x):
if x == 0:
return -50
else:
return ((adc_to_c(x-1)) + 0.05)
def sensor_temp(v):
print(f"SENSOR READ: {v} Volt")
ADC = adc_raw_value(v)
if ADC is None:
print("Voltage is not within the sensor range")
else:
print("**********************\nAnalog to Digital Convertion\n**********************")
print(f"Analog: {v}\nDigital: {ADC}")
print("**********************")
print(f"Temperature is: {round(adc_to_c(ADC))}")
sensor_temp(MIN_VOLT)
SENSOR READ: 0 Volt
**********************
Analog to Digital Convertion
**********************
Analog: 0
Digital: 0
**********************
Temperature is: -50
sensor_temp(MAX_VOLT)
SENSOR READ: 2.442 Volt
**********************
Analog to Digital Convertion
**********************
Analog: 2.442
Digital: 2000
**********************
Temperature is: 50
sensor_temp(1.221)
SENSOR READ: 1.221 Volt
**********************
Analog to Digital Convertion
**********************
Analog: 1.221
Digital: 1000
**********************
Temperature is: 0
sensor_temp(1.5)
SENSOR READ: 1.5 Volt
**********************
Analog to Digital Convertion
**********************
Analog: 1.5
Digital: 1228
**********************
Temperature is: 11
Sorting Numpy Arrays
- Sort a Numpy array by using the inbuilt sort function
import numpy as np
a = np.array([34, 5, 89, 23, 76])
print(np.sort(a))
Output:
[ 5 23 34 76 89]
Story Generator
- Generate random stories using the random module.
import random
when = ['A few years ago', 'Yesterday', 'Last night', 'A long time ago','On 20th Jan']
who = ['a rabbit', 'an elephant', 'a mouse', 'a turtle','a cat']
name = ['Ali', 'Miriam','daniel', 'Hoouk', 'Starwalker']
residence = ['Barcelona','India', 'Germany', 'Venice', 'England']
went = ['cinema', 'university','seminar', 'school', 'laundry']
happened = ['made a lot of friends','Eats a burger', 'found a secret key', 'solved a mystery', 'wrote a book']
print(random.choice(when) + ', ' + random.choice(who) + ' that lived in ' + random.choice(residence) + ', went to the ' + random.choice(went) + ' and ' + random.choice(happened))
Output:
On 20th Jan, a turtle that lived in Venice, went to the university and solved a mystery
Display Calendar
- Display the calendar by importing the calendar module to our program
import calendar
#initialize the year and the month variables
yr = 2024
mnth = 2
# printing the calendar
print(calendar.month(yr, mnth))
Output:
February 2024
Mo Tu We Th Fr Sa Su
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29
Invoice Generator
- An invoice is a bill that serves as proof of a transaction between a buyer and a seller. here, we need only basic print and formatting statements
# create a product and price for three items
product1_name, product1_price = 'Books', 50.95
product2_name, product2_price = 'Computer', 598.99
product3_name, product3_price = 'Monitor', 156.89
# create a company name and information
company_name = 'Thecleverprogrammer, inc.'
company_address = '144 Kalka ji.'
company_city = 'New Delhi'
# declare ending message
message = 'Thanks for shopping with us today!'
# create a top border
print('*' * 50)
# print company information first using format
print('\t\t{}'.format(company_name.title()))
print('\t\t{}'.format(company_address.title()))
print('\t\t{}'.format(company_city.title()))
# print a line between sections
print('=' * 50)
# print out header for section of items
print('\tProduct Name\tProduct Price')
# create a print statement for each item
print('\t{}\t\t${}'.format(product1_name.title(), product1_price))
print('\t{}\t${}'.format(product2_name.title(), product2_price))
print('\t{}\t\t${}'.format(product3_name.title(), product3_price))
# print a line between sections
print('=' * 50)
# print out header for section of total
print('\t\t\tTotal')
# calculate total price and print out
total = product1_price + product2_price + product3_price
print('\t\t\t${}'.format(total))
# print a line between sections
print('=' * 50)
# output thank you message
print('\n\t{}\n'.format(message))
# create a bottom border
print('*' * 50)
Output:
**************************************************
Thecleverprogrammer, Inc.
144 Kalka Ji.
New Delhi
==================================================
Product Name Product Price
Books $50.95
Computer $598.99
Monitor $156.89
==================================================
Total
$806.83
==================================================
Thanks for shopping with us today!
**************************************************
Using Strings
- Working with strings: objects that contain sequences of character data.
name="john"+" "+"smith"
print(name.title())
print("Hello {},you are {} years old".format(name.title(),28))
print(f"Hello {name.title()}")
print("It is {},he is {} years old and his name is {}!".format(False,28,"John Smith".title()))
print(name.replace("john smith","Said ZITOUNI"))
Output:
John Smith
Hello John Smith,you are 28 years old
Hello John Smith
It is False,he is 28 years old and his name is John Smith!
Said ZITOUNI
name="john"+" "+"smith"
print(name.replace("john smith","Said ZITOUNI"))
print(name.upper())
print(name.find("ohn"))
print(name.strip())
list=name.split(" ")
print(list)
s="$$John"
print(s.lstrip('$'))
Output:
Said ZITOUNI
JOHN SMITH
1
john smith
['john', 'smith']
John
- Read more about strings here.
Guess a Word
- The function get_word returns a random word from a list of words
import random
def get_word():
"""
This function returns a random word from a list of words
"""
word_list = ["apple", "banana", "cherry", "date", "elderberry", "fig", "grape"]
return random.choice(word_list)
def play_game():
word = get_word()
word_letters = set(word)
alphabet = set('abcdefghijklmnopqrstuvwxyz')
used_letters = set()
lives = 6
# Loop until either the word is guessed or the lives run out
while len(word_letters) > 0 and lives > 0:
# Display the current state of the word
word_list = [letter if letter in used_letters else '_' for letter in word]
print("Current word: ", ' '.join(word_list))
# Get a user's guess
user_letter = input("Guess a letter: ").lower()
if user_letter in alphabet - used_letters:
used_letters.add(user_letter)
if user_letter in word_letters:
word_letters.remove(user_letter)
else:
lives = lives - 1
print("Letter is not in word.")
elif user_letter in used_letters:
print("You have already used that letter. Guess again.")
else:
print("Invalid character. Please enter a letter.")
# The game has ended, display the result
if lives == 0:
print("You died, sorry. The word was", word)
else:
print("You guessed the word", word, "!!")
if __name__ == '__main__':
play_game()
Output:
Current word: _ _ _ _ _
Guess a letter: a
Current word: _ _ a _ _
Guess a letter: c
Letter is not in word.
Current word: _ _ a _ _
Guess a letter: e
Current word: _ _ a _ e
Guess a letter: g
Current word: g _ a _ e
Guess a letter: r
Current word: g r a _ e
Guess a letter: p
You guessed the word grape !!
Tip Calculator
- Implementing the Tip Calculator
#If the bill was $150.00, split between 5 people, with 12% tip.
#Each person should pay (150.00 / 5) * 1.12 = 33.6
#Format the result to 2 decimal places = 33.60
#Tip: You might need to do some research in Google to figure out how to do this.
print("Welcome to the Tip Calculator")
bill = float(input("What was the total bill? $"))
percentage = int(input("What percentage tip would you like to give? 10, 12, or 15? "))
people_count = int(input("How many people to split the bill? "))
tip = bill * (percentage / 100)
total_bill = bill + tip
individual_amount = total_bill / people_count
final_amount = format(individual_amount, '.2f')
print(f"Each person should pay ${final_amount}")
- Example I/O
Welcome to the Tip Calculator
What was the total bill? $150
What percentage tip would you like to give? 10, 12, or 15? 12
How many people to split the bill? 5
Each person should pay $33.60
Pizza Deliveries
- Implementing the Pizza Deliveries chatbot
print("===================================")
print("WELCOME TO PYTHON PIZZA DELIVERIES!")
print("===================================\n")
total_bill = 0
def pizza_size():
small_pizza = 15
medium_pizza = 20
large_pizza = 25
global total_bill
size = str(input("What size pizza do you want? S, M, or L ")).upper()
if size == "S":
total_bill += small_pizza
print("You want a small pizza.")
print("-----------------------\n")
pepperoni(size)
elif size == "M":
total_bill += medium_pizza
print("You want a medium pizza.")
print("------------------------\n")
pepperoni(size)
elif size == "L":
total_bill += large_pizza
print("You want a large pizza.")
print("-----------------------\n")
pepperoni(size)
else:
print("Please respond with S, M, or L")
print("------------------------------\n")
pizza_size()
def pepperoni(size):
sml_pep = 2
med_lar_pep = 3
global total_bill
add_pepperoni = str(input("Do you want pepperoni? Y or N ")).upper()
if size == "S" and add_pepperoni == "Y":
total_bill += sml_pep
print("You want pepperoni.")
print("-------------------\n")
extra_cheese()
elif (size == "M" or "L") and add_pepperoni == "Y":
total_bill += med_lar_pep
print("You want pepperoni.")
print("-------------------\n")
extra_cheese()
elif add_pepperoni == "N":
print("You don't want pepperoni.")
print("-------------------------\n")
extra_cheese()
else:
print("Please respond with Y or N")
print("--------------------------\n")
pepperoni(size)
def extra_cheese():
add_cheese = 1
global total_bill
more_cheese = str(input("Do you want extra cheese? Y or N ")).upper()
if more_cheese == "Y":
total_bill += add_cheese
print("You want extra cheese.")
print("----------------------\n")
print(f"Your final bill is: ${total_bill}.")
elif more_cheese == "N":
print("You don't want extra cheese.")
print("----------------------------\n")
print(f"Your final bill is: ${total_bill}.")
else:
print("Please respond with Y or N")
print("--------------------------\n")
extra_cheese()
pizza_size()
- Example I/O
===================================
WELCOME TO PYTHON PIZZA DELIVERIES!
===================================
What size pizza do you want? S, M, or L L
You want a large pizza.
-----------------------
Do you want pepperoni? Y or N Y
You want pepperoni.
-------------------
Do you want extra cheese? Y or N Y
You want extra cheese.
----------------------
Your final bill is: $29.
Highest Score
- Calculating the highest student scores from a list of students
# HIGHEST STUDENT SCORES
# Example input = 67 82 98 67 82
student_scores = input("Input a list of student scores ").split()
for n in range(0, len(student_scores)):
student_scores[n] = int(student_scores[n])
print(student_scores)
# Method 1 - Easy
highest_score = 0
for score in student_scores:
if score > highest_score:
highest_score = score
print(f"The highest score in the class is: {highest_score}")
- Example I/O
Input a list of student scores 22 45 67 82 44
[22, 45, 67, 82, 44]
The highest score in the class is: 82
Password Generator
- Implementing a random password generator
#Password Generator Project
import random
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o',
'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D',
'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S',
'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
numbers = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']
symbols = ['!', '#', '$', '%', '&', '(', ')', '*', '+']
print("Welcome to the PyPassword Generator!")
nr_letters= int(input("How many letters would you like in your password?\n"))
nr_symbols = int(input(f"How many symbols would you like?\n"))
nr_numbers = int(input(f"How many numbers would you like?\n"))
pw_list = []
for i in range(0, nr_letters):
pw_list.append(random.choice(letters))
for i in range(0, nr_symbols):
pw_list.append(random.choice(symbols))
for i in range(0, nr_numbers):
pw_list.append(random.choice(numbers))
random.shuffle(pw_list)
final_pw = ''.join(pw_list)
print(f"Your password is: {final_pw}")
- Example I/O
Welcome to the PyPassword Generator!
How many letters would you like in your password?
8
How many symbols would you like?
5
How many numbers would you like?
3
Your password is: )SDniE*34+&PcU1*
Paint Area Calculator
- Program which calculates how many cans of paint are needed to paint a wall (1 can of paint can cover 5 square meters of a wall)
import math
# 1 can of paint can cover 5 square meters of a wall.
def paint_calc(height, width, cover):
number_of_cans = int(math.ceil((height * width) / cover))
print(f"You'll need {number_of_cans} cans of paint.")
test_h = int(input("Height of wall: "))
test_w = int(input("Width of wall: "))
coverage = 5
paint_calc(height=test_h, width=test_w, cover=coverage)
- Example I/O
Height of wall: 3
Width of wall: 5
You'll need 3 cans of paint.
Menu-Driven Program
- Implementing a menu-driven program for students who are willing to take admitted to a university called PrepBytes University.
def cse():
print("You will get the following subjects")
print("DSA")
print("DBMS")
print("Computer Networks (CN)")
def it():
print("You will get the following subjects")
print("DSA")
print("DBMS")
print("Computer Networks (CN)")
print("MPMC (Multi processor and Multi Controller)")
print("Information Security")
def ece():
print("You will get the following subjects")
print("Wireless Communication")
print("Switching Theory and Logic Design (STLD)")
print("Mobile Systems Communication")
def ee():
print("You will get the following subjects")
print("Switching Theory and Logic Design (STLD)")
print("Control Systems")
print("Electrical Circuits and Designs")
def mech():
print("You will get the following subjects")
print("Force and Friction")
print("Motors and its Types")
def main():
print("Welcome to PrepBytes University Portal!!!")
print("Select one of the following streams in which you want to study")
while(True):
print("1. Computer Science")
print("2. Information Technology")
print("3. Electronics and Communication")
print("4. Electrical and Electronics")
print("5. Mechanical")
print("10. Exit")
print("Enter the choice number from above")
choice = int(input())
if choice == 1:
print("You have chosen Computer Science.");
cse();
print("Are you sure you want to lock this stream? Choose either A or B.")
print("A. Yes, I'm sure")
print("B. No, I'm not sure")
ch = input()[0]
if ch == 'A':
print("Congratulations!! You are now a CSE student at PrepBytes");
break
elif choice == 2:
print("You have chosen Information Technology.");
it();
print("Are you sure you want to lock this stream? Choose either A or B.")
print("A. Yes, I'm sure")
print("B. No, I'm not sure")
ch = input()[0]
if ch == 'A':
print("Congratulations!! You are now an IT student at PrepBytes");
break
elif choice == 3:
print("You have chosen Electronics and Communication.");
ece();
print("Are you sure you want to lock this stream? Choose either A or B.")
print("A. Yes, I'm sure")
print("B. No, I'm not sure")
ch = input()[0]
if ch == 'A':
print("Congratulations!! You are now an ECE student at PrepBytes");
break
elif choice == 4:
print("You have chosen Electrical and Electronics.");
ee();
print("Are you sure you want to lock this stream? Choose either A or B.")
print("A. Yes, I'm sure")
print("B. No, I'm not sure")
ch = input()[0]
if ch == 'A':
print("Congratulations!! You are now an EE student at PrepBytes");
break
elif choice == 5:
print("You have chosen Mechanical Engineering.");
mech();
print("Are you sure you want to lock this stream? Choose either A or B.")
print("A. Yes, I'm sure")
print("B. No, I'm not sure")
ch = input()[0]
if ch == 'A':
print("Congratulations!! You are now a ME student at PrepBytes");
break
elif choice == 10:
break
else:
print("You have entered an invalid choice.")
if __name__ == '__main__':
main()
- Example I/O
Welcome to PrepBytes University Portal!!!
Select one of the following streams in which you want to study
1. Computer Science
2. Information Technology
3. Electronics and Communication
4. Electrical and Electronics
5. Mechanical
10. Exit
Enter the choice number from above
1
You have chosen Computer Science.
You will get the following subjects
DSA
DBMS
Computer Networks (CN)
Are you sure you want to lock this stream? Choose either A or B.
A. Yes, I'm sure
B. No, I'm not sure
A
Congratulations!! You are now a CSE student at PrepBytes
Datetime Module
- Exploring the datetime module
import datetime
# Create a date object for January 1, 2023
d = datetime.date(2023, 1, 1)
print(d)
# Get the current date
today = datetime.date.today()
print(today)
# Get the year, month, and day of a date object
year = today.year
month = today.month
day = today.day
print(year, month, day) # print each value separately
# Format a date object as a string
date_string = today.strftime("%d/%m/%Y")
print(date_string)
# Parse a date string into a date object
date_string = "2023-03-15"
parsed_date = datetime.datetime.strptime(date_string, "%Y-%m-%d").date()
print(parsed_date)
# Get the day of the week as an integer (Monday is 0 and Sunday is 6)
weekday = today.weekday()
print(weekday)
- Output:
2023-01-01
2024-02-20
2024 2 20
20/02/2024
2023-03-15
1
- Let’s take an example of the time class
import datetime
# Create a time object for 9:30 AM
t = datetime.time(9, 30)
print(t)
# Get the current time
now = datetime.datetime.now()
current_time = now.time()
print(current_time)
# Get the hour, minute, and second of a time object
hour = t.hour
minute = t.minute
second = t.second
print(hour, minute, second) # print each value separately
# Format a time object as a string
time_string = t.strftime("%I:%M %p")
print(time_string)
# Compare two time objects
time1 = datetime.time(8, 30)
time2 = datetime.time(9, 30)
if time1 < time2:
print("time1 is earlier than time2")
else:
print("time1 is late than time2")
- Output:
09:30:00
21:25:29.116006
9 30 0
09:30 AM
time1 is earlier than time2
Counting Digits
- Program for counting the digits in a number
num=int(input("Enter number: "))
count=0
i = num
# count the digits
while(i>0):
count=count+1
i=i//10
print(f"The number of digits in {num}:",count)
- Example I/O
Enter number: 1000
The number of digits in 1000: 4
Largest Number
- Program for finding the largest number in a list
mylist = [101, 62, 66, 45, 99, 18, 266, 18]
maxi = mylist[0] # initializing maximum value
for i in mylist:
if i > maxi:
maxi = i
# printing the largest number
print("Largest number is:", maxi)
Output:
Largest number is: 266
Join Two Strings
x = "Programming"
y = "Languages"
z = x + y
print(z)
Output:
ProgrammingLanguages
Format Floating Point in the String
- Format floating point in the string using String Formatting
# Use of String Formatting
x = 462.75897
print("{:5.2f}".format(x))
# Use of String Interpolation
y = 462.75897
print("%5.2f" % y)
Output:
462.76
462.76
Raise a Number to a Power
- Raise a number to a power using math
import math
# Assign values to a and n
a = 4
n = 3
# Method 1
b = a ** n
print("%d to the power %d is %d" % (a,n,b))
# Method 2
b = pow(a,n)
print("%d to the power %d is %d" % (a,n,b))
# Method 3
b = math.pow(a,n)
print("%d to the power %d is %5.2f" % (a,n,b))
Output:
4 to the power 3 is 64
4 to the power 3 is 64
4 to the power 3 is 64.00
Working with Boolean Types
# Boolean value
x = True
print(x)
# Number to Boolean
x = 10
print(bool(x))
x = -5
print(bool(x))
x = 0
print(bool(x))
# Boolean from comparison operator
x = 6
y = 3
print(x < y)
Output:
True
True
True
False
False
If Else Statement
- Example of the If Else Statement
# Assign a numeric value
x = 35
# Check the is more than 35 or not
if (x >= 35):
print("You have passed")
else:
print("You have not passed")
Output:
You have passed
Using AND/OR Operators
- Example of using AND and OR operators
# Take practical marks
x = float(input("Enter the Practical marks: "))
# Take theory marks
y = float(input("Enter the Theory marks: "))
# Check the passing condition using AND and OR operator
if (x >= 25 and y >= 45) or (x + y) >=70:
print("\nYou have passed")
else:
print("\nYou have failed")
- Example I/O
Enter the Practical marks: 30
Enter the Theory marks: 40
You have passed
Switch Case Statement
- Example of the switch case statement
# Switcher for implementing switch case options
def employee_details(ID):
switcher = {
"5006": "Employee Name: John",
"5008": "Employee Name: Ram",
"5010": "Employee Name: Mohamend",
}
'''The first argument will be returned if the match found and
employee ID does not exist will be returned if no match found'''
return switcher.get(ID, "employee ID does not exist")
# Take the employee ID
ID = input("Enter the employee ID: ")
# Print the output
print(employee_details(ID))
Output:
Enter the employee ID: 5008
Employee Name: Ram
While Loop
- Example of While Loop
# Initialize counter
counter = 1
# Iterate the loop 9 times
while counter < 10:
# Print the counter value
print ("%d" % counter)
# Increment the counter
counter = counter + 1
Output:
1
2
3
4
5
6
7
8
9
Use of regex
- Example of using regex
# Import re module
import re
# Take any string data
string = input("Enter a string value: ")
# Define the searching pattern
pattern = 'Ale'
# match the pattern with input value
found = re.match(pattern, string)
# Print message based on the return value
if found:
print("The input value is started with the capital letter")
else:
print("You have to type string start with the capital letter")
- Example I/O
Enter a string value: Alessandro
The input value is started with the capital letter
Use of getpass
- Example of using getpass
# import getpass module
import getpass
# Take password from the user
passwd = getpass.getpass('Password:')
# Check the password
if passwd == "python":
print("You are verified")
else:
print("You are not verified")
- Example I/O
Password:········
You are verified
Use of Date Format
- Example of using date format
from datetime import date
# Read the current date
current_date = date.today()
# Print the formatted date
print("Today is :%d-%d-%d" % (current_date.day,current_date.month,current_date.year))
# Set the custom date
custom_date = date(2026, 12, 26)
print("The date is:",custom_date)
Output:
Today is :23-2-2024
The date is: 2026-12-26
Add/Remove the Item from a List
# Declare a fruit list
fruits = ["Mango","Orange","Guava","Banana"]
# Insert an item in the 2nd position
fruits.insert(1, "Apple")
# Displaying list after inserting
print("The fruit list after insert:")
print(fruits)
# Remove an item
fruits.remove("Banana")
# Print the list after delete
print("The fruit list after delete:")
print(fruits)
Output:
The fruit list after insert:
['Mango', 'Apple', 'Orange', 'Guava', 'Banana']
The fruit list after delete:
['Mango', 'Apple', 'Orange', 'Guava']
Slice Data
- Slice a string using 1, 2, and 3 parameters
# Assign string value
text = "Python Programming Language"
# Slice using one parameter
sliceObj = slice(5)
print(text[sliceObj])
# Slice using two parameter
sliceObj = slice(6,12)
print(text[sliceObj])
# Slice using three parameter
sliceObj = slice(6,25,5)
print(text[sliceObj])
Output:
Pytho
Progr
rnn
Add and Search Data in the Set
# Define the number set
numbers = {13, 10, 56, 18, 12, 44, 87}
# Add a new data
numbers.add(63)
# Print the set values
print(numbers)
message = "Number is not found"
# Take a number value for search
search_number = int(input("Enter a number:"))
# Search the number in the set
for val in numbers:
if val == search_number:
message = "Number is found"
break
print(message)
- Example I/O
{18, 87, 56, 10, 12, 13, 44, 63}
Enter a number:10
Number is found
Count Items in the List
- Count items in the list
# Define the string
string = 'Python Go JavaScript HTML CSS MYSQL Python'
# Define the search string
search = 'Python'
# Store the count value
count = string.count(search)
# Print the formatted output
print("%s appears %d times" % (search, count))
Output:
Python appears 2 times
Define and Call a Function
- Example of using functions
# Define addition function
def addition(number1, number2):
result = number1 + number2
print("Addition result:",result)
# Define area function with return statement
def area(radius):
result = 3.14 * radius * radius
return result
# Call addition function
addition(5, 3)
# Call area function
print("Area of the circle is",area(2))
Output:
Addition result: 8
Area of the circle is 12.56
Using Try-Except Blocks
- Example of using try-except blocks
# Try block
try:
# Take a number
number = int(input("Enter a number: "))
if number % 2 == 0:
print("Number is even")
else:
print("Number is odd")
# Exception block
except (ValueError):
# Print error message
print("Enter a numeric value")
- Examples I/O
Enter a number: 16
Number is even
Enter a number: 7
Number is odd
Enter a number: a1
Enter a numeric value
Read/Write Files
- Example of reading and writing files
#Assign the filename
filename = "names.txt"
# Open file for writing
fileHandler = open(filename, "w")
# Add some text
fileHandler.write("Ram\n")
fileHandler.write("John\n")
fileHandler.write("David\n")
# Close the file
fileHandler.close()
# Open file for reading
fileHandler = open(filename, "r")
# Read a file line by line
for line in fileHandler:
print(line)
# Close the file
fileHandler.close()
Output:
Ram
John
David
List Files in a Directory
- Example to print the content of the directory YOURPATH
# Import os module to read directory
import os
# Set the directory path
path = 'YOURPATH'
# Read the content of the file
files = os.listdir(path)
# Print the content of the directory
for file in files:
print(file)
- Example I/O
alzheimerfeatures.txt
amazon_cells_labelled.txt
Automated_Responsive_Search_Ads.csv
ballonboys.PNG
ballongirl.PNG
ballononly.PNG
ballonwoman.PNG
ballooninput.PNG
balloononlyinput.PNG
balloontop.PNG
banking_finance_insurance_ml_overview.txt
bank_marketing_report.pdf
beginneroverview.txt
car data.csv
CAR DETAILS FROM CAR DEKHO.csv
Read/Write w/ pickle
- Example of using pickle
# Import pickle module
import pickle
# Declare the object to store data
dataObject = []
# Iterate the for loop for 5 times
for num in range(10,15):
dataObject.append(num)
# Open a file for writing data
file_handler = open('numbers', 'wb')
# Dump the data of the object into the file
pickle.dump(dataObject, file_handler)
# close the file handler
file_handler.close()
# Open a file for reading the file
file_handler = open('numbers', 'rb')
# Load the data from the file after deserialization
dataObject = pickle.load(file_handler)
# Iterate the loop to print the data
for val in dataObject:
print(val)
# close the file handler
file_handler.close()
Output:
file numbers
10
11
12
13
14
Use of range Function
- Example of using range() with 1, 2 parameters
# range() with one parameter
for val in range(7):
print(val, end=' ')
print('\n')
# range() with two parameters
for val in range(7,16):
print(val, end=' ')
print('\n')
Output:
0 1 2 3 4 5 6
7 8 9 10 11 12 13 14 15
Use of map Function
- Example of calculating the x to the power n using map()
# Define the function to calculate power
def cal_power(n):
return x ** n
# Take the value of x
x = int(input("Enter the value of x:"))
# Define a tuple of numbers
numbers = [2, 6, 3]
# Calculate the x to the power n using map()
result = map(cal_power, numbers)
print(list(result))
- Example I/O
Enter the value of x:3
[9, 729, 27]
Use of filter Function
- Example of using filter function
# Define a list of participant
participant = ['Ram', 'John', 'David', 'Krishna', 'Prasad']
# Define the function to filters selected candidates
def SelectedPerson(participant):
selected = ['John', 'Rahman', 'Ram']
if(participant in selected):
return True
selectedList = filter(SelectedPerson, participant)
print('The selected candidates are:')
for candidate in selectedList:
print(candidate)
Output:
The selected candidates are:
Ram
John
Pandas First Program
- Example of creating a pandas series
#importing pandas
import pandas as pd
#creating list
data=["Harry","Sam","Juliet","Robert","Max"]
#creating pandas Series
df=pd.Series(data)
#printing Series
print(df)
Output:
0 Harry
1 Sam
2 Juliet
3 Robert
4 Max
dtype: object
Current Weather
- Find current weather of any city using OpenWeatherMap API with your API key
# importing requests and json
import requests, json
# base URL
BASE_URL = "https://api.openweathermap.org/data/2.5/weather?"
#City Name
CITY = "Amsterdam"
# API key
API_KEY = "YOUR_API_KEY"
# upadting the URL
URL = BASE_URL + "q=" + CITY + "&appid=" + API_KEY
# HTTP request
response = requests.get(URL)
# checking the status code of the request
if response.status_code == 200:
# getting data in the json format
data = response.json()
# getting the main dict block
main = data['main']
# getting temperature
temperature = main['temp']-273.15
# getting the humidity
humidity = main['humidity']
# getting the pressure
pressure = main['pressure']
# weather report
report = data['weather']
print(f"{CITY:-^30}")
print(f"Temperature: {temperature}")
print(f"Humidity: {humidity}")
print(f"Pressure: {pressure}")
print(f"Weather Report: {report[0]['description']}")
else:
# showing the error message
print("Error in the HTTP request")
- Example I/O
----------Amsterdam-----------
Temperature: 9.720000000000027
Humidity: 82
Pressure: 1020
Weather Report: broken clouds
Turtle Race Game
- This code introduces for loops through a fun turtle race game. Loops are used to draw the race track and to make the turtles move a random number of steps each turn. If you have a group of people to play the game, each person pick a turtle and the one that gets the furthest is the winner.
from turtle import Turtle, Screen
import random
is_race_on = False
screen = Screen()
screen.setup(width=500, height=400)
user_bet = screen.textinput(title="Make your bet", prompt="Which turtle will win the race? Enter a color:")
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
y_positions = [-70, -40, -10, 20, 50, 80]
all_turtles = []
for turtle_index in range(0, 6):
new_turtle = Turtle(shape="turtle")
new_turtle.color(colors[turtle_index])
new_turtle.penup()
new_turtle.goto(x=-230, y=y_positions[turtle_index])
all_turtles.append(new_turtle)
if user_bet:
is_race_on = True
while is_race_on:
for turtle in all_turtles:
if turtle.xcor() > 230:
is_race_on = False
winning_turtle = turtle.pencolor()
if winning_turtle == user_bet:
print(f"You've won! The {winning_turtle} is the winner!")
else:
print(f"You've lost! The {winning_turtle} is the winner!")
rand_distance = random.randint(0, 10)
turtle.forward(rand_distance)
screen.exitonclick()
- Example I/O
Make your bet
Which turtle will win the race? Enter a color:
red
You've lost! The blue is the winner!
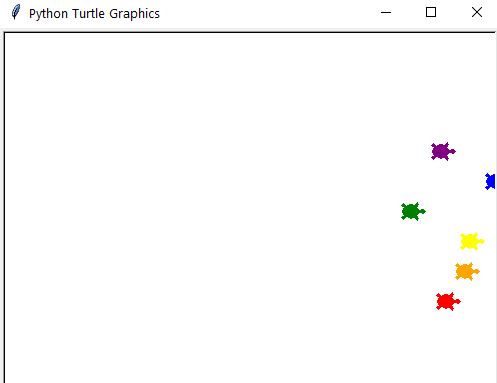
Must Watch Movie List
- Creating the 100 Must Watch Movie List using web scraping on a movie website with BeautifulSoup
import requests
from bs4 import BeautifulSoup
URL = "https://web.archive.org/web/20200518073855/https://www.empireonline.com/movies/features/best-movies-2/"
response = requests.get(URL)
response_text = response.text
soup = BeautifulSoup(response_text, 'html.parser')
movies = []
titles = soup.find_all(name='h3', class_='title')
for i in titles:
with open('movies.txt', 'a',encoding="utf-8" ) as file:
file.write(i.getText()+"\n")
- Example I/O: the file movies.txt containing the list of 100 must watch movies, viz.
100) Stand By Me
99) Raging Bull
98) Amelie
97) Titanic
96) Good Will Hunting
95) Arrival
94) Lost In Translation
93) The Princess Bride
92) The Terminator
91) The Prestige
90) No Country For Old Men
89) Shaun Of The Dead
88) The Exorcist
87) Predator
86) Indiana Jones And The Last Crusade
etc.
Digital Clock
- Creating a digital clock using Tkinter
from tkinter import *
import time
root = Tk()
root.title('Digital Clock')
root.geometry('300x100')
clock_label = Label(root, font=('Arial', 30, 'bold'),
bg='black', fg='white', bd=30)
clock_label.pack(fill=BOTH, expand=1)
def update_clock():
current_time = time.strftime('%H:%M:%S')
clock_label.config(text=current_time)
clock_label.after(1000, update_clock)
update_clock()
root.mainloop()
- Example I/O
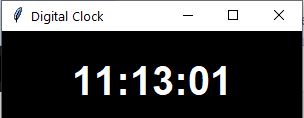
BMI Calculator
- Creating the BMI Calculator
Height = float(input('Enter your Height in centimeters: '))
Weight = float(input('Enter your Weight in Kg: '))
Height = Height/100
BMI = Weight/(Height*Height)
print('your Body Mass Index is: ', BMI)
if(BMI > 0):
if(BMI <= 16):
print('you are severely underweight')
elif(BMI <= 18.5):
print('you are underweight')
elif(BMI <= 25):
print('you are Healthy')
elif(BMI <= 30):
print('you are overweight')
else:
print('you are severely overweight')
else:
print('Enter valid details')
- Example I/O
Enter your Height in centimeters: 180
Enter your Weight in Kg: 90
your Body Mass Index is: 27.777777777777775
you are overweight
YouTube Downloader
- Creating the YouTube downloader using pytube
!pip install pytube
Successfully installed pytube-15.0.0
from pytube import YouTube
link = input('https://youtu.be/GtvUxXyFoMw')
yt = YouTube(link)
yt.streams.first().download()
print('download', link)
- Example I/O
Input the link:
https://youtu.be/GtvUxXyFoMw
download https://youtu.be/GtvUxXyFoMw
- Output mp4 file (5.4 Mb)
How to become a software engineer Important tips to become a software engineer
Factorial of a Number
- Defining the function factorial_of_number
def factorial_of_number(n):
if n < 0:
raise ValueError("The Number you entered is less than 0")
if n is 1:
return 1
elif n is 0:
return 1
else:
return n * factorial_of_number(n-1)
- Example I/O
print(factorial_of_number(6))
720
Numpy Linear Algebra
- Let’s learn how to use Numpy to work with numerical data
#Import Statement
import numpy as np
#1-Dimensional Arrays (Vectors)
my_array = np.array([1.1, 9.2, 8.1, 4.7])
my_array.shape
(4,)
my_array[2]
8.1
my_array.ndim
1
#2-Dimensional Arrays (Matrices)
array_2d = np.array([[1, 2, 3, 9],
[5, 6, 7, 8]])
array_2d[0, :]
array([1, 2, 3, 9])
mystery_array = np.array([[[0, 1, 2, 3],
[4, 5, 6, 7]],
[[7, 86, 6, 98],
[5, 1, 0, 4]],
[[5, 36, 32, 48],
[97, 0, 27, 18]]])
# Note all the square brackets!
mystery_array.ndim
3
mystery_array.shape
(3, 2, 4)
mystery_array[2, 1, 3]
18
mystery_array[:, :, 0]
#Use .arange()to createa a vector a with values ranging from 10 to 29.
a = np.arange(10, 30)
print(a)
10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29]
#Use Python slicing techniques
a[-3:]
array([27, 28, 29])
a[3:6]
array([13, 14, 15])
a[12:]
array([22, 23, 24, 25, 26, 27, 28, 29])
a[::2]
array([10, 12, 14, 16, 18, 20, 22, 24, 26, 28])
#Reverse the order of the values in a, so that the first element comes last
np.flip(a)
array([29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13,
12, 11, 10])
#Linear Algebra with Vectors
v1 = np.array([4, 5, 2, 7])
v2 = np.array([2, 1, 3, 3])
print(v1 + v2)
[ 6 6 5 10]
#Scalars
array2d = np.array([[1, 2, 3, 4], [5, 6, 7, 8]])
array2d + 10
array([[11, 12, 13, 14],
[15, 16, 17, 18]])
array2d * 5
array([[ 5, 10, 15, 20],
[25, 30, 35, 40]])
#Matrix Multiplication
a1 = np.array([[1, 3],
[0, 1],
[6, 2],
[9, 7]])
b1 = np.array([[4, 1, 3],
[5, 8, 5]])
np.matmul(a1, b1)
array([[19, 25, 18],
[ 5, 8, 5],
[34, 22, 28],
[71, 65, 62]])
a1 @ b1
array([[19, 25, 18],
[ 5, 8, 5],
[34, 22, 28],
[71, 65, 62]])
#Use the .random() function
from numpy.random import random
random((3, 3, 3))
array([[[0.13277451, 0.56517025, 0.39505373],
[0.72784423, 0.33856398, 0.40790094],
[0.45777358, 0.80555047, 0.9188987 ]],
[[0.21392271, 0.31767031, 0.35165927],
[0.07417224, 0.23895659, 0.98454047],
[0.22460087, 0.10410174, 0.11090475]],
[[0.26892163, 0.98770413, 0.43427793],
[0.8109698 , 0.52696712, 0.40471147],
[0.63502182, 0.24586102, 0.94698587]]])
#Use the .linspace() function
x = np.linspace(0, 100, num=9)
print(x)
x.shape
[ 0. 12.5 25. 37.5 50. 62.5 75. 87.5 100. ]
(9,)
Numpy/Matplotlib Images
- Manipulating Images as ndarrays and plotting with Matplotlib
!pip install scipy, matplotlib
from scipy import ndimage, misc
from matplotlib import pyplot as plt
img1 = misc.face()
plt.imshow(img1)

type(img1)
numpy.ndarray
img1.shape
(768, 1024, 3)
img1.ndim
3
#Image rotation
plt.imshow(np.rot90(img1))
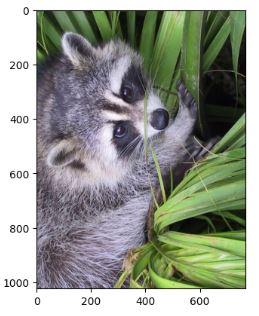
#Solar image
solar_img = 255 - img1
plt.imshow(solar_img)
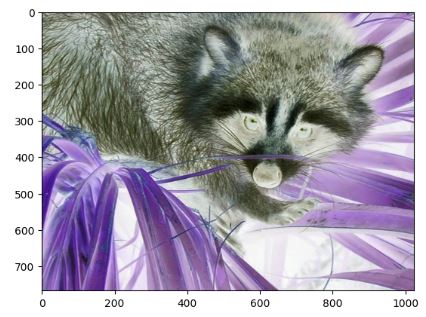
#Image conversion to the gray scale
grey_vals = np.array([0.2126, 0.7152, 0.0722])
sRGB_array = img1/ 255
img_grey = sRGB_array @ grey_vals
plt.imshow(img_grey, cmap='gray')
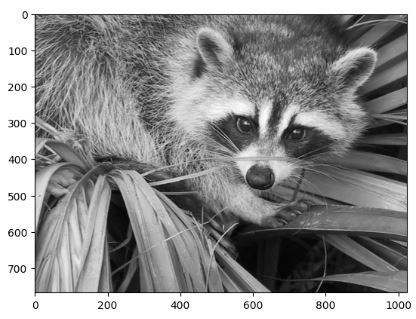
# Image flip
plt.imshow(np.flip(img_grey), cmap='gray')
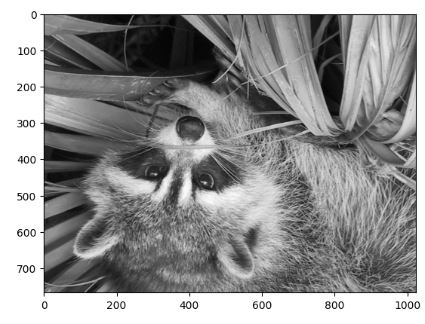
Numpy Financial Module
- Within the realm of Numpy, the
numpy-financial
module serves as a valuable tool set for anyone dealing with financial mathematics. This module extends Numpy’s capabilities by offering financial functions commonly used in banking, investment, and other financial sectors. - Installing the module with pip
!pip install numpy-financial
- Using IRR to calculate the annual rate of return of an investment
import numpy_financial as npf
npf.irr([-750500, 45000, 150700, 700000, 220000, 307000])
Output:
0.21287538856102062
- Using the Time Value of Money (TVM) Functions
import numpy_financial as npf
# Define variables
rate = 0.05 # Interest rate (5%)
nper = 10 # Number of periods
pmt = -1000 # Periodic payment (negative for outgoing cash flow)
pv = 0 # Present value or initial investment
# Calculate Future Value (FV)
fv = npf.fv(rate, nper, pmt, pv)
print(f"Future Value (FV): ${fv:.2f}")
# Calculate Present Value (PV)
pv = npf.pv(rate, nper, pmt, fv)
print(f"Present Value (PV): ${pv:.2f}")
# Calculate Number of Payment Periods (NPER)
nper = npf.nper(rate, pmt, pv, fv)
print(f"Number of Payment Periods (NPER): {nper:.2f} periods")
# Calculate Periodic Payment (PMT)
pmt = npf.pmt(rate, nper, pv, fv)
print(f"Periodic Payment (PMT): ${pmt:.2f}")
Output:
Future Value (FV): $12577.89
Present Value (PV): $-0.00
Number of Payment Periods (NPER): 10.00 periods
Periodic Payment (PMT): $-1000.00
- Using Net Present Value (NPV) and Internal Rate of Return (IRR)
import numpy_financial as npf
# Define the cash flows (negative values for investments, positive for returns)
cash_flows = [-1000, 300, 300, 300, 300]
# Define the discount rate (rate of return)
discount_rate = 0.1 # 10%
# Calculate NPV
npv = npf.npv(discount_rate, cash_flows)
print(f"Net Present Value (NPV): {npv:.2f}")
# Calculate IRR
irr = npf.irr(cash_flows)
print(f"Internal Rate of Return (IRR): {irr:.2%}")
Output:
Net Present Value (NPV): -49.04
Internal Rate of Return (IRR): 7.71%
- Depreciation Calculations:
- Straight-line depreciation and double-declining balance depreciation are two methods of calculating depreciation for assets.
- Straight-line depreciation is the simplest method, and it allocates an equal amount of depreciation expense to each year of the asset’s useful life.
- Double-declining balance depreciation depreciates an asset more rapidly in the early years of its useful life. It calculates the depreciation expense as a multiple of the straight-line depreciation expense.
- We use these methods to allocate the cost of an asset over its useful life. This is done for financial reporting purposes, to match the expenses of using the asset with the revenues generated by the asset.
def straight_line_depreciation(cost, salvage_value, useful_life):
annual_depreciation = (cost - salvage_value) / useful_life
return annual_depreciation
def double_declining_balance_depreciation(cost, salvage_value, useful_life, period):
depreciation_rate = 2 / useful_life
previous_period_depreciation = 0
for _ in range(period):
current_period_depreciation = (cost - previous_period_depreciation) * depreciation_rate
previous_period_depreciation += current_period_depreciation
return min(current_period_depreciation, cost - salvage_value)
# Example usage
cost = 10000 # Initial cost of the asset
salvage_value = 2000 # Estimated salvage value at the end of its useful life
useful_life = 5 # Number of years the asset will be used
period = 3 # The specific year for which you want to calculate depreciation
depreciation = double_declining_balance_depreciation(cost, salvage_value, useful_life, period)
print(f"Depreciation in year {period}: ${depreciation:.2f}")
depreciation = straight_line_depreciation(cost, salvage_value, useful_life)
print(f"Annual Depreciation: ${depreciation:.2f}")
Output:
Depreciation in year 3: $1440.00
Annual Depreciation: $1600.00
- These functions are useful for asset depreciation and tax planning.
- Rate of Return Calculations, viz. the Modified Internal Rate of Return (MIRR) and the Internal Rate of Return (XIRR)
import numpy_financial as npf
from pyxirr import xirr
import pandas as pd
from datetime import date
# Cash flows for the investment or project
cash_flows = [-1000, 200, 250, 300, 350]
dates = [date(2019, 1, 1),date(2020, 1, 1), date(2021, 1, 1), date(2022, 1, 1), date(2023, 1, 1)]
# Finance rate (cost of capital)
finance_rate = 0.1
# Reinvestment rate (rate at which positive cash flows are reinvested)
reinvest_rate = 0.12
# feed columnar data
xirr(dates, cash_flows)
# feed tuples
xirr(zip(dates, cash_flows))
# Calculate the Modified Internal Rate of Return (MIRR)
mirr = npf.mirr(cash_flows, finance_rate, reinvest_rate)
# Calculate the Internal Rate of Return (XIRR)
xirr = xirr(pd.DataFrame({"dates": dates, "cash_flows": cash_flows}))
print(f"The Modified Internal Rate of Return (MIRR) is: {mirr:.2%}")
print(f"The Internal Rate of Return (XIRR) is: {xirr:.2%}")
Output:
The Modified Internal Rate of Return (MIRR) is: 6.38%
The Internal Rate of Return (XIRR) is: 3.58%
Creating Pandas Data Objects
- Pandas provides two types of data objects:
- Creating a Series
import pandas as pd
import numpy as np
s = pd.Series([1, 3, 5, np.nan, 6, 8])
s
0 1.0
1 3.0
2 5.0
3 NaN
4 6.0
5 8.0
dtype: float64
- Creating a DataFrame
data = {
'apples': [3, 2, 0, 1],
'oranges': [0, 3, 7, 2]
}
purchases = pd.DataFrame(data)
purchases
apples oranges
0 3 0
1 2 3
2 0 7
3 1 2
- Read more here.
I/O Pandas DataFrames
df = pd.DataFrame(np.random.randint(0, 5, (10, 5)))
df.to_csv("tmp.csv")
dfin=pd.read_csv("tmp.csv")
dfin
Unnamed: 0 0 1 2 3 4
0 0 3 0 1 4 1
1 1 0 3 3 0 0
2 2 0 1 3 1 4
3 3 3 2 0 4 0
4 4 0 3 0 1 4
5 5 4 0 3 4 3
6 6 1 0 3 4 3
7 7 3 2 0 2 4
8 8 4 2 0 3 2
9 9 2 1 2 0 1
Tkinter Sentiment Detector GUI
- Creating a Sentiment Detector GUI application using Tkinter
!pip install vaderSentiment
# import SentimentIntensityAnalyzer class
# from vaderSentiment.vaderSentiment module.
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
# import all methods and classes from the tkinter
from tkinter import *
# Function for clearing the
# contents of all entry boxes
# And text area.
def clearAll() :
# deleting the content from the entry box
negativeField.delete(0, END)
neutralField.delete(0, END)
positiveField.delete(0, END)
overallField.delete(0, END)
# whole content of text area is deleted
textArea.delete(1.0, END)
# function to print sentiments
# of the sentence.
def detect_sentiment():
# get a whole input content from text box
sentence = textArea.get("1.0", "end")
# Create a SentimentIntensityAnalyzer object.
sid_obj = SentimentIntensityAnalyzer()
# polarity_scores method of SentimentIntensityAnalyzer
# object gives a sentiment dictionary.
# which contains pos, neg, neu, and compound scores.
sentiment_dict = sid_obj.polarity_scores(sentence)
string = str(sentiment_dict['neg']*100) + "% Negative"
negativeField.insert(10, string)
string = str(sentiment_dict['neu']*100) + "% Neutral"
neutralField.insert(10, string)
string = str(sentiment_dict['pos']*100) +"% Positive"
positiveField.insert(10, string)
# decide sentiment as positive, negative and neutral
if sentiment_dict['compound'] >= 0.05 :
string = "Positive"
elif sentiment_dict['compound'] <= - 0.05 :
string = "Negative"
else :
string = "Neutral"
overallField.insert(10, string)
# Driver Code
if __name__ == "__main__" :
# Create a GUI window
gui = Tk()
# Set the background colour of GUI window
gui.config(background = "light green")
# set the name of tkinter GUI window
gui.title("Sentiment Detector")
# Set the configuration of GUI window
gui.geometry("250x400")
# create a label : Enter Your Task
enterText = Label(gui, text = "Enter Your Sentence",
bg = "light green")
# create a text area for the root
# with lunida 13 font
# text area is for writing the content
textArea = Text(gui, height = 5, width = 25, font = "lucida 13")
# create a Submit Button and place into the root window
# when user press the button, the command or
# function affiliated to that button is executed
check = Button(gui, text = "Check Sentiment", fg = "Black",
bg = "Red", command = detect_sentiment)
# Create a negative : label
negative = Label(gui, text = "sentence was rated as: ",
bg = "light green")
# Create a neutral : label
neutral = Label(gui, text = "sentence was rated as: ",
bg = "light green")
# Create a positive : label
positive = Label(gui, text = "sentence was rated as: ",
bg = "light green")
# Create a overall : label
overall = Label(gui, text = "Sentence Overall Rated As: ",
bg = "light green")
# create a text entry box
negativeField = Entry(gui)
# create a text entry box
neutralField = Entry(gui)
# create a text entry box
positiveField = Entry(gui)
# create a text entry box
overallField = Entry(gui)
# create a Clear Button and place into the root window
# when user press the button, the command or
# function affiliated to that button is executed .
clear = Button(gui, text = "Clear", fg = "Black",
bg = "Red", command = clearAll)
# create a Exit Button and place into the root window
# when user press the button, the command or
# function affiliated to that button is executed .
Exit = Button(gui, text = "Exit", fg = "Black",
bg = "Red", command = exit)
# grid method is used for placing
# the widgets at respective positions
# in table like structure.
enterText.grid(row = 0, column = 2)
textArea.grid(row = 1, column = 2, padx = 10, sticky = W)
check.grid(row = 2, column = 2)
negative.grid(row = 3, column = 2)
neutral.grid(row = 5, column = 2)
positive.grid(row = 7, column = 2)
overall.grid(row = 9, column = 2)
negativeField.grid(row = 4, column = 2)
neutralField.grid(row = 6, column = 2)
positiveField.grid(row = 8, column = 2)
overallField.grid(row = 10, column = 2)
clear.grid(row = 11, column = 2)
Exit.grid(row = 12, column = 2)
# start the GUI
gui.mainloop()
- Example I/O
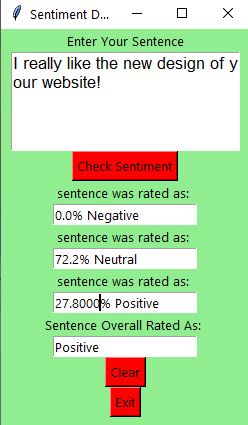
Tkinter/Pillow Slideshow
- Creating a slideshow application with Tkinter and Pillow
# import required modules
import tkinter as tk
from tkinter import *
from PIL import Image
from PIL import ImageTk
# adjust window
root=tk.Tk()
root.geometry("200x200")
# loading the images
img=ImageTk.PhotoImage(Image.open("github2_languages.jpg"))
img2=ImageTk.PhotoImage(Image.open("python_statista_popular.jpeg"))
img3=ImageTk.PhotoImage(Image.open("sentiment_gui.jpg"))
l=Label()
l.pack()
# using recursion to slide to next image
x = 1
# function to change to next image
def move():
global x
if x == 4:
x = 1
if x == 1:
l.config(image=img)
elif x == 2:
l.config(image=img2)
elif x == 3:
l.config(image=img3)
x = x+1
root.after(2000, move)
# calling the function
move()
root.mainloop()
- Example Output
Slideshow Image 1:
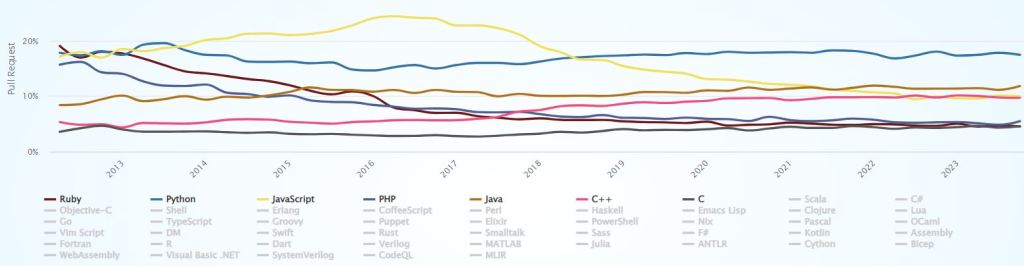
Slideshow Image 2:
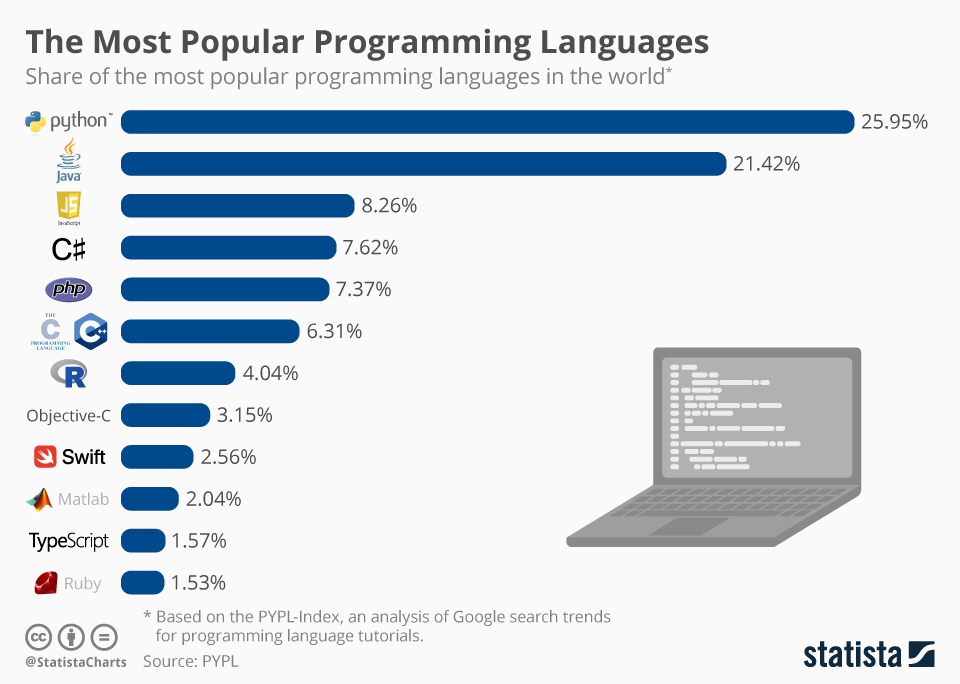
Slideshow Image 3:
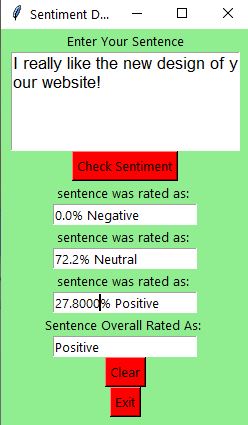
IceCream Debugger
!pip install icecream
from icecream import ic
def func(num):
return num * 2
ic(func(3))
6
sample_dict = {1:"A", 2:"B", 3:"C"}
ic(sample_dict[1])
'A'
def func(num):
ic(num)
return 2*num
func(2)
4
Using Pandas DataFrames
- Pandas is a high-level data manipulation tool. It is built on the Numpy package and its key data structure is called the DataFrame.
- There are several ways to create a DataFrame:
dict = {"country": ["Brazil", "Russia", "India", "China", "South Africa"],
"capital": ["Brasilia", "Moscow", "New Dehli", "Beijing", "Pretoria"],
"area": [8.516, 17.10, 3.286, 9.597, 1.221],
"population": [200.4, 143.5, 1252, 1357, 52.98] }
import pandas as pd
brics = pd.DataFrame(dict)
print(brics)
area capital country population
0 8.516 Brasilia Brazil 200.40
1 17.100 Moscow Russia 143.50
2 3.286 New Dehli India 1252.00
3 9.597 Beijing China 1357.00
4 1.221 Pretoria South Africa 52.98
# Set the index for brics
brics.index = ["BR", "RU", "IN", "CH", "SA"]
# Print out brics with new index values
print(brics)
output:
area capital country population
BR 8.516 Brasilia Brazil 200.40
RU 17.100 Moscow Russia 143.50
IN 3.286 New Dehli India 1252.00
CH 9.597 Beijing China 1357.00
SA 1.221 Pretoria South Africa 52.98
- You can use square brackets to select one column of the
cars
DataFrame
# Import pandas and cars.csv
import pandas as pd
cars = pd.read_csv('cars.csv', index_col = 0)
# Print out country column as Pandas Series
print(cars['cars_per_cap'])
# Print out country column as Pandas DataFrame
print(cars[['cars_per_cap']])
# Print out DataFrame with country and drives_right columns
print(cars[['cars_per_cap', 'country']])
output:
US 809
AUS 731
JAP 588
IN 18
RU 200
MOR 70
EG 45
Name: cars_per_cap, dtype: int64
cars_per_cap
US 809
AUS 731
JAP 588
IN 18
RU 200
MOR 70
EG 45
cars_per_cap country
US 809 United States
AUS 731 Australia
JAP 588 Japan
IN 18 India
RU 200 Russia
MOR 70 Morocco
EG 45 Egypt
- Square brackets can also be used to access observations (rows) from a DataFrame
# Import cars data
import pandas as pd
cars = pd.read_csv('cars.csv', index_col = 0)
# Print out first 4 observations
print(cars[0:4])
# Print out fifth and sixth observation
print(cars[4:6])
output:
cars_per_cap country drives_right
US 809 United States True
AUS 731 Australia False
JAP 588 Japan False
IN 18 India False
cars_per_cap country drives_right
RU 200 Russia True
MOR 70 Morocco True
- Let’s look at a 10 minutes introduction to Pandas, geared mainly for new users. You can see more complex recipes in the Cookbook.
import numpy as np
import pandas as pd
df2 = pd.DataFrame(
{
"A": 1.0,
"B": pd.Timestamp("20130102"),
"C": pd.Series(1, index=list(range(4)), dtype="float32"),
"D": np.array([3] * 4, dtype="int32"),
"E": pd.Categorical(["test", "train", "test", "train"]),
"F": "foo"
}
)
df2
A B C D E F
0 1.0 2013-01-02 1.0 3 test foo
1 1.0 2013-01-02 1.0 3 train foo
2 1.0 2013-01-02 1.0 3 test foo
3 1.0 2013-01-02 1.0 3 train foo
- Viewing data
dates = pd.date_range("20130101", periods=6)
df = pd.DataFrame(np.random.randn(6, 4), index=dates, columns=list("ABCD"))
df
A B C D
2013-01-01 -0.532411 0.067493 -2.355388 1.078265
2013-01-02 -0.884475 1.040327 0.683065 0.160037
2013-01-03 -0.065658 -0.244594 1.065679 0.534151
2013-01-04 -0.986462 -0.103097 -1.458458 1.465258
2013-01-05 1.414690 -0.748344 2.699952 -0.380150
2013-01-06 -0.063310 -0.430136 2.227652 -0.013623
df.head(3)
A B C D
2013-01-01 -0.532411 0.067493 -2.355388 1.078265
2013-01-02 -0.884475 1.040327 0.683065 0.160037
2013-01-03 -0.065658 -0.244594 1.065679 0.534151
df.tail(3)
A B C D
2013-01-04 -0.986462 -0.103097 -1.458458 1.465258
2013-01-05 1.414690 -0.748344 2.699952 -0.380150
2013-01-06 -0.063310 -0.430136 2.227652 -0.013623
describe()
shows a quick statistic summary of your data:
df.describe()
A B C D
count 6.000000 6.000000 6.000000 6.000000
mean -0.186271 -0.069725 0.477084 0.473990
std 0.876396 0.612072 2.008169 0.695308
min -0.986462 -0.748344 -2.355388 -0.380150
25% -0.796459 -0.383751 -0.923077 0.029792
50% -0.299035 -0.173846 0.874372 0.347094
75% -0.063897 0.024846 1.937159 0.942237
max 1.414690 1.040327 2.699952 1.465258
DataFrame.sort_index()
sorts by an axis:
df.sort_index(axis=1, ascending=False)
D C B A
2013-01-01 1.078265 -2.355388 0.067493 -0.532411
2013-01-02 0.160037 0.683065 1.040327 -0.884475
2013-01-03 0.534151 1.065679 -0.244594 -0.065658
2013-01-04 1.465258 -1.458458 -0.103097 -0.986462
2013-01-05 -0.380150 2.699952 -0.748344 1.414690
2013-01-06 -0.013623 2.227652 -0.430136 -0.063310
DataFrame.sort_values()
sorts by values:
df.sort_values(by="B")
A B C D
2013-01-05 1.414690 -0.748344 2.699952 -0.380150
2013-01-06 -0.063310 -0.430136 2.227652 -0.013623
2013-01-03 -0.065658 -0.244594 1.065679 0.534151
2013-01-04 -0.986462 -0.103097 -1.458458 1.465258
2013-01-01 -0.532411 0.067493 -2.355388 1.078265
2013-01-02 -0.884475 1.040327 0.683065 0.160037
Tkinter Calendar
- Creating a Tkinter-based Calendar application that can show the calendar with respect to the given year, given by the user
# import all methods and classes from the tkinter
from tkinter import *
# import calendar module
import calendar
# Function for showing the calendar of the given year
def showCal() :
# Create a GUI window
new_gui = Tk()
# Set the background colour of GUI window
new_gui.config(background = "white")
# set the name of tkinter GUI window
new_gui.title("CALENDAR")
# Set the configuration of GUI window
new_gui.geometry("550x600")
# get method returns current text as string
fetch_year = int(year_field.get())
# calendar method of calendar module return
# the calendar of the given year .
cal_content = calendar.calendar(fetch_year)
# Create a label for showing the content of the calendar
cal_year = Label(new_gui, text = cal_content, font = "Consolas 10 bold")
# grid method is used for placing
# the widgets at respective positions
# in table like structure.
cal_year.grid(row = 5, column = 1, padx = 20)
# start the GUI
new_gui.mainloop()
# Driver Code
if __name__ == "__main__" :
# Create a GUI window
gui = Tk()
# Set the background colour of GUI window
gui.config(background = "white")
# set the name of tkinter GUI window
gui.title("CALENDAR")
# Set the configuration of GUI window
gui.geometry("250x140")
# Create a CALENDAR : label with specified font and size
cal = Label(gui, text = "CALENDAR", bg = "dark gray",
font = ("times", 28, 'bold'))
# Create a Enter Year : label
year = Label(gui, text = "Enter Year", bg = "light green")
# Create a text entry box for filling or typing the information.
year_field = Entry(gui)
# Create a Show Calendar Button and attached to showCal function
Show = Button(gui, text = "Show Calendar", fg = "Black",
bg = "Red", command = showCal)
# Create a Exit Button and attached to exit function
Exit = Button(gui, text = "Exit", fg = "Black", bg = "Red", command = exit)
# grid method is used for placing
# the widgets at respective positions
# in table like structure.
cal.grid(row = 1, column = 1)
year.grid(row = 2, column = 1)
year_field.grid(row = 3, column = 1)
Show.grid(row = 4, column = 1)
Exit.grid(row = 6, column = 1)
# start the GUI
gui.mainloop()
- Example I/O
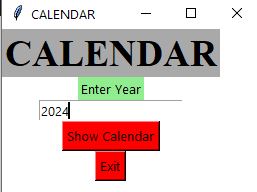
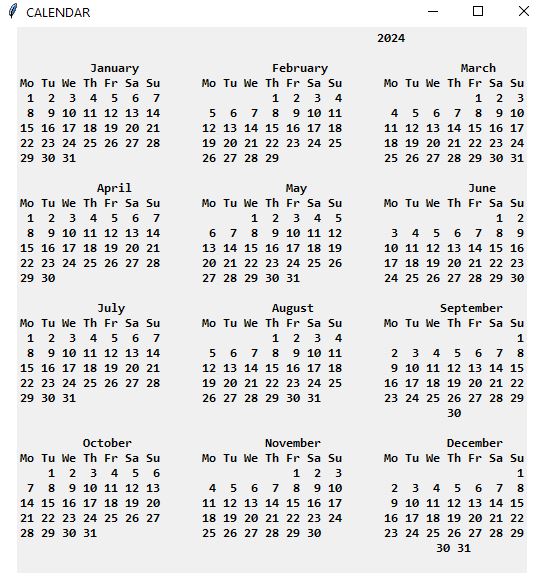
Loan Calculator GUI
- Creating a loan calculator using Tkinter. The calculator will be capable of calculating the total amount and monthly payment based on the loan amount, period and interest rate.
# Import tkinter
from tkinter import *
class LoanCalculator:
def __init__(self):
window = Tk() # Create a window
window.title("Loan Calculator") # Set title
# create the input boxes.
Label(window, text = "Annual Interest Rate").grid(row = 1,
column = 1, sticky = W)
Label(window, text = "Number of Years").grid(row = 2,
column = 1, sticky = W)
Label(window, text = "Loan Amount").grid(row = 3,
column = 1, sticky = W)
Label(window, text = "Monthly Payment").grid(row = 4,
column = 1, sticky = W)
Label(window, text = "Total Payment").grid(row = 5,
column = 1, sticky = W)
# for taking inputs
self.annualInterestRateVar = StringVar()
Entry(window, textvariable = self.annualInterestRateVar,
justify = RIGHT).grid(row = 1, column = 2)
self.numberOfYearsVar = StringVar()
Entry(window, textvariable = self.numberOfYearsVar,
justify = RIGHT).grid(row = 2, column = 2)
self.loanAmountVar = StringVar()
Entry(window, textvariable = self.loanAmountVar,
justify = RIGHT).grid(row = 3, column = 2)
self.monthlyPaymentVar = StringVar()
lblMonthlyPayment = Label(window, textvariable =
self.monthlyPaymentVar).grid(row = 4,
column = 2, sticky = E)
self.totalPaymentVar = StringVar()
lblTotalPayment = Label(window, textvariable =
self.totalPaymentVar).grid(row = 5,
column = 2, sticky = E)
# create the button
btComputePayment = Button(window, text = "Compute Payment",
command = self.computePayment).grid(
row = 6, column = 2, sticky = E)
window.mainloop() # Create an event loop
# compute the total payment.
def computePayment(self):
monthlyPayment = self.getMonthlyPayment(
float(self.loanAmountVar.get()),
float(self.annualInterestRateVar.get()) / 1200,
int(self.numberOfYearsVar.get()))
self.monthlyPaymentVar.set(format(monthlyPayment, '10.2f'))
totalPayment = float(self.monthlyPaymentVar.get()) * 12 \
* int(self.numberOfYearsVar.get())
self.totalPaymentVar.set(format(totalPayment, '10.2f'))
def getMonthlyPayment(self, loanAmount, monthlyInterestRate, numberOfYears):
# compute the monthly payment.
monthlyPayment = loanAmount * monthlyInterestRate / (1
- 1 / (1 + monthlyInterestRate) ** (numberOfYears * 12))
return monthlyPayment;
root = Tk() # create the widget
# call the class to run the program.
LoanCalculator()
- Example I/O

Weight Converter GUI
- Using Tkinter to create a weight converter GUI that accepts a kilogram input value and converts that value to grams, pounds, and ounces when the user clicks the Convert button.
# Python program to create a simple GUI
# weight converter using Tkinter
from tkinter import *
# Create a GUI window
window = Tk()
# Function to convert weight
# given in kg to grams, pounds
# and ounces
def from_kg():
# convert kg to gram
gram = float(e2_value.get())*1000
# convert kg to pound
pound = float(e2_value.get())*2.20462
# convert kg to ounce
ounce = float(e2_value.get())*35.274
# Enters the converted weight to
# the text widget
t1.delete("1.0", END)
t1.insert(END,gram)
t2.delete("1.0", END)
t2.insert(END,pound)
t3.delete("1.0", END)
t3.insert(END,ounce)
# Create the Label widgets
e1 = Label(window, text = "Enter the weight in Kg")
e2_value = StringVar()
e2 = Entry(window, textvariable = e2_value)
e3 = Label(window, text = 'Gram')
e4 = Label(window, text = 'Pounds')
e5 = Label(window, text = 'Ounce')
# Create the Text Widgets
t1 = Text(window, height = 1, width = 20)
t2 = Text(window, height = 1, width = 20)
t3 = Text(window, height = 1, width = 20)
# Create the Button Widget
b1 = Button(window, text = "Convert", command = from_kg)
# grid method is used for placing
# the widgets at respective positions
# in table like structure
e1.grid(row = 0, column = 0)
e2.grid(row = 0, column = 1)
e3.grid(row = 1, column = 0)
e4.grid(row = 1, column = 1)
e5.grid(row = 1, column = 2)
t1.grid(row = 2, column = 0)
t2.grid(row = 2, column = 1)
t3.grid(row = 2, column = 2)
b1.grid(row = 0, column = 2)
# Start the GUI
window.mainloop()
- Example I/O

Age Calculator GUI
- Creating a Tkinter based simple Age Calculator application that can calculate the age with respect to the given date and birth date, given by the user.
import all functions from the tkinter
from tkinter import *
# import messagebox class from tkinter
from tkinter import messagebox
# Function for clearing the
# contents of all text entry boxes
def clearAll() :
# deleting the content from the entry box
dayField.delete(0, END)
monthField.delete(0, END)
yearField.delete(0, END)
givenDayField.delete(0, END)
givenMonthField.delete(0, END)
givenYearField.delete(0, END)
rsltDayField.delete(0, END)
rsltMonthField.delete(0, END)
rsltYearField.delete(0, END)
# function for checking error
def checkError() :
# if any of the entry field is empty
# then show an error message and clear
# all the entries
if (dayField.get() == "" or monthField.get() == ""
or yearField.get() == "" or givenDayField.get() == ""
or givenMonthField.get() == "" or givenYearField.get() == "") :
# show the error message
messagebox.showerror("Input Error")
# clearAll function calling
clearAll()
return -1
# function to calculate Age
def calculateAge() :
# check for error
value = checkError()
# if error is occur then return
if value == -1 :
return
else :
# take a value from the respective entry boxes
# get method returns current text as string
birth_day = int(dayField.get())
birth_month = int(monthField.get())
birth_year = int(yearField.get())
given_day = int(givenDayField.get())
given_month = int(givenMonthField.get())
given_year = int(givenYearField.get())
# if birth date is greater then given birth_month
# then donot count this month and add 30 to the date so
# as to subtract the date and get the remaining days
month =[31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if (birth_day > given_day):
given_month = given_month - 1
given_day = given_day + month[birth_month-1]
# if birth month exceeds given month, then
# donot count this year and add 12 to the
# month so that we can subtract and find out
# the difference
if (birth_month > given_month):
given_year = given_year - 1
given_month = given_month + 12
# calculate day, month, year
calculated_day = given_day - birth_day;
calculated_month = given_month - birth_month;
calculated_year = given_year - birth_year;
# calculated day, month, year write back
# to the respective entry boxes
# insert method inserting the
# value in the text entry box.
rsltDayField.insert(10, str(calculated_day))
rsltMonthField.insert(10, str(calculated_month))
rsltYearField.insert(10, str(calculated_year))
# Driver Code
if __name__ == "__main__" :
# Create a GUI window
gui = Tk()
# Set the background colour of GUI window
gui.configure(background = "light green")
# set the name of tkinter GUI window
gui.title("Age Calculator")
# Set the configuration of GUI window
gui.geometry("525x260")
# Create a Date Of Birth : label
dob = Label(gui, text = "Date Of Birth", bg = "blue")
# Create a Given Date : label
givenDate = Label(gui, text = "Given Date", bg = "blue")
# Create a Day : label
day = Label(gui, text = "Day", bg = "light green")
# Create a Month : label
month = Label(gui, text = "Month", bg = "light green")
# Create a Year : label
year = Label(gui, text = "Year", bg = "light green")
# Create a Given Day : label
givenDay = Label(gui, text = "Given Day", bg = "light green")
# Create a Given Month : label
givenMonth = Label(gui, text = "Given Month", bg = "light green")
# Create a Given Year : label
givenYear = Label(gui, text = "Given Year", bg = "light green")
# Create a Years : label
rsltYear = Label(gui, text = "Years", bg = "light green")
# Create a Months : label
rsltMonth = Label(gui, text = "Months", bg = "light green")
# Create a Days : label
rsltDay = Label(gui, text = "Days", bg = "light green")
# Create a Resultant Age Button and attached to calculateAge function
resultantAge = Button(gui, text = "Resultant Age", fg = "Black", bg = "Red", command = calculateAge)
# Create a Clear All Button and attached to clearAll function
clearAllEntry = Button(gui, text = "Clear All", fg = "Black", bg = "Red", command = clearAll)
# Create a text entry box for filling or typing the information.
dayField = Entry(gui)
monthField = Entry(gui)
yearField = Entry(gui)
givenDayField = Entry(gui)
givenMonthField = Entry(gui)
givenYearField = Entry(gui)
rsltYearField = Entry(gui)
rsltMonthField = Entry(gui)
rsltDayField = Entry(gui)
# grid method is used for placing
# the widgets at respective positions
# in table like structure .
dob.grid(row = 0, column = 1)
day.grid(row = 1, column = 0)
dayField.grid(row = 1, column = 1)
month.grid(row = 2, column = 0)
monthField.grid(row = 2, column = 1)
year.grid(row = 3, column = 0)
yearField.grid(row = 3, column = 1)
givenDate.grid(row = 0, column = 4)
givenDay.grid(row = 1, column = 3)
givenDayField.grid(row = 1, column = 4)
givenMonth.grid(row = 2, column = 3)
givenMonthField.grid(row = 2, column = 4)
givenYear.grid(row = 3, column = 3)
givenYearField.grid(row = 3, column = 4)
resultantAge.grid(row = 4, column = 2)
rsltYear.grid(row = 5, column = 2)
rsltYearField.grid(row = 6, column = 2)
rsltMonth.grid(row = 7, column = 2)
rsltMonthField.grid(row = 8, column = 2)
rsltDay.grid(row = 9, column = 2)
rsltDayField.grid(row = 10, column = 2)
clearAllEntry.grid(row = 12, column = 2)
# Start the GUI
gui.mainloop()
- Example I/O
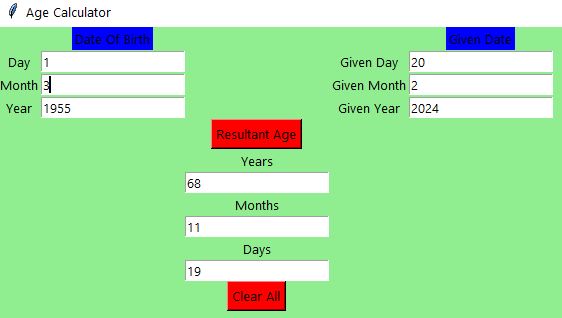
Create Dictionary from an Object
- This is a Python Program to form a dictionary from an object of a class
class A(object):
def __init__(self):
self.A=1
self.B=2
obj=A()
print(obj.__dict__)
Output:
{'A': 1, 'B': 2}
Check a Key in a Dictionary
- This is a code to check if a given key exists in a dictionary or not
d={'A':1,'B':2,'C':3}
key=input("Enter key to check:")
if key in d.keys():
print("Key is present and value of the key is:")
print(d[key])
else:
print("Key isn't present!")
- Examples I/O
Enter key to check:B
Key is present and value of the key is:
2
Enter key to check:D
Key isn't present!
Add a Key-Value Pair to the Dictionary
- Example to add a key-value pair to the dictionary
key=int(input("Enter the key (int) to be added:"))
value=int(input("Enter the value for the key to be added:"))
d={}
d.update({key:value})
print("Updated dictionary is:")
print(d)
- Example I/O
Enter the key (int) to be added:6
Enter the value for the key to be added:666
Updated dictionary is:
{6: 666}
Iterate Over Dictionaries Using for Loop
dt = {'a': 'juice', 'b': 'grill', 'c': 'corn'}
for key, value in dt.items():
print(key, value)
Output:
a juice
b grill
c corn
Check the File Size
- Using os module to Check the File Size
import os
file_stat = os.stat('ballonboys.png')
print(file_stat.st_size)
Output:
188858
(188.8Kb)
Working with Functions
- A function is a block of code which only runs when it is called. As a Data Scientist, you’ll constantly need to write your own functions to solve problems that your data poses to you:
# setting default parameter values
def calcArea(r, pi=3.14):
area = pi*(r**2)
print( "Area: {}".format(area))
calcArea(2) # assuming radius is the value of 2
Area: 12.56
Output:
Area: 12.56
# using args parameter to take in a tuple of arbitrary values
def outputData(name,*args):
print(type(args))
print(args)
for arg in args:
print(arg)
outputData("John Smith",5, True, "Jess",123)
Output:
<class 'tuple'>
(5, True, 'Jess', 123)
5
True
Jess
123
# using kwargs parameter to take in a dictionary of arbitrary values
def outputData(**kwargs):
print( type(kwargs))
print(kwargs)
print( kwargs[ "name" ] )
print( kwargs[ "num" ] )
outputData(name = "John Smith", num = 5, b = True)
Output:
<class 'dict'>
{'name': 'John Smith', 'num': 5, 'b': True}
John Smith
5
Working with Dictionaries
- Dictionaries are used to store data values in key:value pairs.
empty={}
person= {'name':'John','age':26}
print(person)
print(dict())
print(person["name"],person["age"])
print(person.get("birthday","birthday is not available"))
# improperly storing a list within a dictionary
sports = [ "baseball", "football", "hockey", "soccer" ]
sports_dict = {"sports":sports} # will produce error, no key
print(sports_dict)
Output:
{'name': 'John', 'age': 26}
{}
John 26
birthday is not available
{'sports': ['baseball', 'football', 'hockey', 'soccer']}
# updating a value for a key/value pair that already exists
car = { "year": 2018, "color": "Blue" }
car["color"] = "White"
print( "Year: {} \t Color: {}".format( car["year"], car["color"] ) )
# deleting a key/value pair from a dictionary
car = { "year": 2018,"model":"Mercedes" }
try:
del car["year"]
print(car)
except:
print("That key does not exist")
Output:
Year: 2018 Color: White
{'model': 'Mercedes'}
Remove First N Characters from a String
- An example of removing characters from a string starting from zero up to
n
and return a new string
def remove_chars(word, n):
print('Original string:', word)
x = word[n:]
return x
print("Removing characters from a string")
print(remove_chars("pynative", 4))
print(remove_chars("pynative", 2))
Output:
Removing characters from a string
Original string: pynative
tive
Original string: pynative
native
Working with Classes
class Car():
color="red"
sound="beep"
ford=Car()
print(ford.color)
print(ford.sound)
ford.sound="honk"
print(ford.sound)
print(ford)
red
beep
honk
class Car():
def __init__(self,color,year):
self.color=color
self.year=year
ford=Car("Blue",2008)
renault=Car("Grey",2011)
print(ford.color,ford.year)
print(renault.color,renault.year)
Blue 2008
Grey 2011
# using and accessing global class attributes
class Car( ):
sound = "beep" # global attribute, accessible through the class itself
def __init__(self, color):
self.color = "blue" # instance specific attribute, not accessible through the class itself
print(Car.sound)
ford = Car("blue")
print(ford.sound, ford.color)
ford.sound="honk"
print(ford.sound)
beep
beep blue
honk
class Dog():
species="Canine"
def __init__(self,name,breed):
self.name=name
self.breed=breed
dog1=Dog("Sammi","Husky")
dog2=Dog("Casey","Chocolate Lab")
print("My dog is from {} species. His name is {} and his breed is {}.".format(dog1.species,dog1.name,dog1.breed))
My dog is from Canine species. His name is Sammi and his breed is Husky.
class Person():
name=input("please type your name: ")
x=Person()
print(x.name)
please type your name: Al
Al
class Dog:
def MakeSound(self):
print("Bark!")
sam=Dog()
sam.MakeSound()
Bark!
# understanding which methods are accessible via the class itself and class instances
class Dog( ):
sound = "bark"
def makeSound(self):
print(self.sound)
def printInfo( ):
print("I am a dog.")
Dog.printInfo( )
sam = Dog()
sam.makeSound()
I am a dog.
bark
class Dog():
def ShowAge(self,age):
print(age)
sam=Dog()
sam.ShowAge(6)
6
# using methods to set or return attribute values, proper programming practice
class Dog( ):
name = ' ' # would normally use init method to declare, this is for testing purposes
def setName(self, new_name):
self.name = new_name # declares the new value for the name attribute
def getName(self):
return self.name # returns the value of the name attribute
sam = Dog( )
sam.setName("Sammi")
print( sam.getName( ) ) # prints the returned value of self.name
Sammi
class Dog():
age=5
def HappyBirthday(self):
self.age+=1
sam=Dog()
for i in range(27):
sam.HappyBirthday()
print(sam.age)
32
class Dog():
age = 6
def GetAge(self):
return self.age
def PrintInfo(self):
if self.GetAge()<10:
print("Puppy!")
sam=Dog()
print(sam.GetAge())
sam.PrintInfo()
6
Puppy!
class Duck:
def __init__(self,**kwargs):
self.variables=kwargs
def set_variable(self,k,v):
self.variables[k]=v
def get_variable(self,k):
return self.variables.get(k,None)
def main():
donald = Duck(feet=52,color='red')
print(donald.get_variable('feet'))
print(donald.get_variable('color'))
donald.set_variable('color','blue')
donald.set_variable('IQ','low')
print(donald.get_variable('IQ'))
if __name__ == "__main__": main()
52
red
low
Define Class and Method
- Example defining the class/method, creating the object, and calling the class method
# Define the class
class Employee:
name = "Johnson"
# Define the method
def details(self):
print("Post: Associate")
print("Department: QC")
print("Salary: $6000")
# Create the employee object
emp = Employee()
# Print the class variable
print("Name:",emp.name)
# Call the class method
emp.details()
Output:
Name: Johnson
Post: Associate
Department: QC
Salary: $6000
List Operations via Classes
- Example to append, delete and display elements of a list using classes
class check():
def __init__(self):
self.n=[]
def add(self,a):
return self.n.append(a)
def remove(self,b):
self.n.remove(b)
def dis(self):
return (self.n)
obj=check()
choice=1
while choice!=0:
print("0. Exit")
print("1. Add")
print("2. Delete")
print("3. Display")
choice=int(input("Enter choice: "))
if choice==1:
n=int(input("Enter number to append: "))
obj.add(n)
print("List: ",obj.dis())
elif choice==2:
n=int(input("Enter number to remove: "))
obj.remove(n)
print("List: ",obj.dis())
elif choice==3:
print("List: ",obj.dis())
elif choice==0:
print("Exiting!")
else:
print("Invalid choice!!")
print()
- Example I/O
0. Exit
1. Add
2. Delete
3. Display
Enter choice: 1
Enter number to append: 4
List: [4]
0. Exit
1. Add
2. Delete
3. Display
Enter choice: 3
List: [4]
0. Exit
1. Add
2. Delete
3. Display
Enter choice: 2
Enter number to remove: 4
List: []
0. Exit
1. Add
2. Delete
3. Display
Enter choice: 0
Exiting!
Minimize Lateness
- Example to minimize maximum lateness using the greedy algorithm
def minimize_lateness(ttimes, dtimes):
"""Return minimum max lateness and the schedule to obtain it.
(min_lateness, schedule) is returned.
Lateness of a request i is L(i) = finish time of i - deadline of if
request i finishes after its deadline.
The maximum lateness is the maximum value of L(i) over all i.
min_lateness is the minimum value of the maximum lateness that can be
achieved by optimally scheduling the requests.
schedule is a list that contains the indexes of the requests ordered such
that minimum maximum lateness is achieved.
ttime[i] is the time taken to complete request i.
dtime[i] is the deadline of request i.
"""
# index = [0, 1, 2, ..., n - 1] for n requests
index = list(range(len(dtimes)))
# sort according to deadlines
index.sort(key=lambda i: dtimes[i])
min_lateness = 0
start_time = 0
for i in index:
min_lateness = max(min_lateness,
(ttimes[i] + start_time) - dtimes[i])
start_time += ttimes[i]
return min_lateness, index
n = int(input('Enter number of requests: '))
ttimes = input('Enter the time taken to complete the {} request(s) in order: '
.format(n)).split()
ttimes = [int(tt) for tt in ttimes]
dtimes = input('Enter the deadlines of the {} request(s) in order: '
.format(n)).split()
dtimes = [int(dt) for dt in dtimes]
min_lateness, schedule = minimize_lateness(ttimes, dtimes)
print('The minimum maximum lateness:', min_lateness)
print('The order in which the requests should be scheduled:', schedule)
- Example I/O
Enter number of requests: 3
Enter the time taken to complete the 3 request(s) in order: 3 4 5
Enter the deadlines of the 3 request(s) in order: 4 3 1
The minimum maximum lateness: 8
The order in which the requests should be scheduled: [2, 1, 0]
Compute a Polynomial Equation
- Example to compute a polynomial equation given that the coefficients of the polynomial are stored in the list
import math
print("Enter the coefficients of the form ax^3 + bx^2 + cx + d")
lst=[]
for i in range(0,4):
a=int(input("Enter coefficient:"))
lst.append(a)
x=int(input("Enter the value of x:"))
sum1=0
j=3
for i in range(0,3):
while(j>0):
sum1=sum1+(lst[i]*math.pow(x,j))
break
j=j-1
sum1=sum1+lst[3]
print("The value of the polynomial is:",sum1)
- Example I/O
Enter the coefficients of the form ax^3 + bx^2 + cx + d
Enter coefficient:3
Enter coefficient:4
Enter coefficient:1
Enter coefficient:2
Enter the value of x:12
The value of the polynomial is: 5774.0
Creating Linked Lists
- Here is the source code to create a linked list and display its elements
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.last_node = None
def append(self, data):
if self.last_node is None:
self.head = Node(data)
self.last_node = self.head
else:
self.last_node.next = Node(data)
self.last_node = self.last_node.next
def display(self):
current = self.head
while current is not None:
print(current.data, end = ' ')
current = current.next
a_llist = LinkedList()
n = int(input('How many elements would you like to add? '))
for i in range(n):
data = int(input('Enter data item: '))
a_llist.append(data)
print('The linked list: ', end = '')
a_llist.display()
- Example I/O
How many elements would you like to add? 4
Enter data item: 21
Enter data item: 22
Enter data item: 23
Enter data item: 24
The linked list: 21 22 23 24
CockTail Sort Algorithm
- Example to implement the cocktail shaker sort algorithm.
def cocktail_shaker_sort(alist):
def swap(i, j):
alist[i], alist[j] = alist[j], alist[i]
upper = len(alist) - 1
lower = 0
no_swap = False
while (not no_swap and upper - lower > 1):
no_swap = True
for j in range(lower, upper):
if alist[j + 1] < alist[j]:
swap(j + 1, j)
no_swap = False
upper = upper - 1
for j in range(upper, lower, -1):
if alist[j - 1] > alist[j]:
swap(j - 1, j)
no_swap = False
lower = lower + 1
alist = input('Enter the list of numbers: ').split()
alist = [int(x) for x in alist]
cocktail_shaker_sort(alist)
print('Sorted list: ', end='')
print(alist)
- Example I/O
Enter the list of numbers: 1 3 122 33 12 31 67
Sorted list: [1, 3, 12, 31, 33, 67, 122]
Binary Search via Recursion
- Example to implement binary search using recursion
def binary_search(alist, start, end, key):
"""Search key in alist[start... end - 1]."""
if not start < end:
return -1
mid = (start + end)//2
if alist[mid] < key:
return binary_search(alist, mid + 1, end, key)
elif alist[mid] > key:
return binary_search(alist, start, mid, key)
else:
return mid
alist = input('Enter the sorted list of numbers: ')
alist = alist.split()
alist = [int(x) for x in alist]
key = int(input('The number to search for: '))
index = binary_search(alist, 0, len(alist), key)
if index < 0:
print('{} was not found.'.format(key))
else:
print('{} was found at index {}.'.format(key, index))
- Example I/O
Enter the sorted list of numbers: 1 3 12 22 24 29 124 134 168
The number to search for: 22
22 was found at index 3.
Find Simple Interest
- Example calculates simple interest based on the principal amount, interest rate, and time period provided:
- Simple Interest = (P × R × T)/100
Here, P = Principal Amount, R = Rate of Interest and T = Time.
principle=float(input("Enter the principle amount:"))
time=int(input("Enter the time(years):"))
rate=float(input("Enter the rate:"))
simple_interest=(principle*time*rate)/100
print("The simple interest is:",simple_interest)
- Example I/O
Enter the principle amount:1000
Enter the time(years):5
Enter the rate:2
The simple interest is: 100.0
Probability Distributions in SciPy
- Let’s work with probability distributions using
SciPy
#Imports
import scipy.stats as stats
import numpy as np
%matplotlib inline
import matplotlib.pyplot as plt
#Initialize this object to have mean 100 and standard deviation 15
normal=stats.norm(loc=100, scale=15)
#Generate a random sample by calling the method .rvs(size=10)
normal.rvs(size=10,random_state=143)
normal_sample = normal.rvs(size=1000,random_state=123)
#Data visualization
plt.hist(normal_sample,density=True,label="Sample")
plt.title("Normal Distribution")
plt.ylabel("Density")
plt.legend()
plt.show()
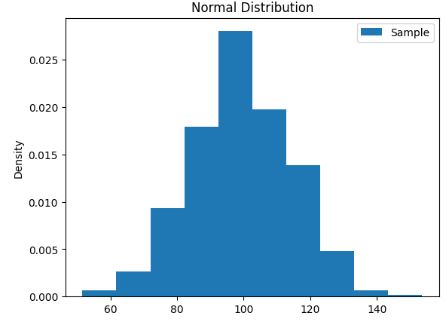
- This is a histogram of 1000 random numbers generated from a normal distribution.
Piecewise Linear 1-D Interpolation in Numpy
- You can use the
numpy.interp
routine to perform a piecewise linear (a.k.a. broken line) interpolation
import numpy as np
#Input data
x = np.linspace(0, 10, num=11)
y = np.cos(-x**2 / 9.0)
#Interpolated data
xnew = np.linspace(0, 10, num=1001)
ynew = np.interp(xnew, x, y)
#Plotting
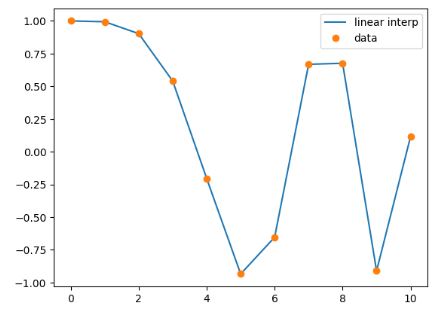
Cubic Spline 1-D Interpolation in Scipy
- To produce a smoother curve, you can use cubic splines in Scipy as follows
!pip install scipy
from scipy.interpolate import CubicSpline
x = np.linspace(0, 10, num=11)
y = np.cos(-x**2 / 9.)
spl = CubicSpline(x, y)
import matplotlib.pyplot as plt
fig, ax = plt.subplots(4, 1, figsize=(5, 7))
xnew = np.linspace(0, 10, num=1001)
ax[0].plot(xnew, spl(xnew))
ax[0].plot(x, y, 'o', label='data')
ax[1].plot(xnew, spl(xnew, nu=1), '--', label='1st derivative')
ax[2].plot(xnew, spl(xnew, nu=2), '--', label='2nd derivative')
ax[3].plot(xnew, spl(xnew, nu=3), '--', label='3rd derivative')
for j in range(4):
ax[j].legend(loc='best')
plt.tight_layout()
plt.show()
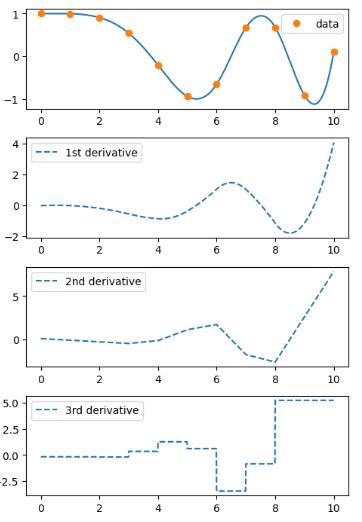
Interpolation with Radial Basis Function
- Interpolate following xs and ys using rbf and find values for 2.1, 2.2 … 2.9:
from scipy.interpolate import Rbf
import numpy as np
xs = np.arange(10)
ys = xs**2 + np.sin(xs) + 1
interp_func = Rbf(xs, ys)
newarr = interp_func(np.arange(2.1, 3, 0.1))
print(newarr)
Output:
[6.25748981 6.62190817 7.00310702 7.40121814 7.8161443 8.24773402
8.69590519 9.16070828 9.64233874]
SciPy T-Test
- T-tests are used to determine if there is significant deference between means of two variables and lets us know if they belong to the same distribution.
import numpy as np
from scipy.stats import ttest_ind
v1 = np.random.normal(size=100)
v2 = np.random.normal(size=100)
res = ttest_ind(v1, v2)
print(res)
Output:
TtestResult(statistic=-0.8997123569279699, pvalue=0.3693662643518123, df=198.0)
- If you want to return only the p-value, use the
pvalue
property:
res = ttest_ind(v1, v2).pvalue
print(res)
Output:
0.3693662643518123
KS-Test
- KS test is used to check if given values follow a distribution
import numpy as np
from scipy.stats import kstest
v = np.random.normal(size=100)
res = kstest(v, 'norm')
print(res)
Output:
KstestResult(statistic=0.1104241179689904, pvalue=0.1618558205968601, statistic_location=-0.43874235149535057, statistic_sign=-1)
Statistical Description of Data
- In order to see a summary of values in an array, we can use the
describe()
function from scipy.stats
import numpy as np
from scipy.stats import describe
v = np.random.normal(size=100)
res = describe(v)
print(res)
Output:
DescribeResult(nobs=100, minmax=(-2.7571044220956993, 2.544130284926933), mean=-0.0105847884817091, variance=1.1206726848849227, skewness=0.012446214239738313, kurtosis=-0.23013590681590568)
Exporting Data in Matlab Format
- SciPy provides us with interoperability with Matlab. SciPy provides us with the module
scipy.io
, which has functions for working with Matlab arrays.
from scipy import io
import numpy as np
arr = np.arange(10)
io.savemat('arr.mat', {"vec": arr})
- The example above saves a file name “arr.mat” in your working directory.
Import Data from Matlab Format
- The
loadmat()
function allows us to import data from a Matlab file.
from scipy import io
import numpy as np
arr = np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9,])
# Export:
io.savemat('arr.mat', {"vec": arr})
# Import:
mydata = io.loadmat('arr.mat')
print(mydata)
Output:
{'__header__': b'MATLAB 5.0 MAT-file Platform: nt, Created on: Fri Feb 23 22:08:10 2024', '__version__': '1.0', '__globals__': [], 'vec': array([[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]])}
- Use the variable name “vec” to display only the array from the Matlab data:
print(mydata['vec'])
[[0 1 2 3 4 5 6 7 8 9]]
SciPy Optimizers
- Example: Find root of the equation
x + cos(x)
from scipy.optimize import root
from math import cos
def eqn(x):
return x + cos(x)
myroot = root(eqn, 0)
print(myroot.x)
Output:
[-0.73908513]
Working with Spatial Data
- One method to generate these triangulations through points is the
Delaunay()
Triangulation
import numpy as np
from scipy.spatial import Delaunay
import matplotlib.pyplot as plt
points = np.array([
[2, 4],
[3, 4],
[3, 0],
[2, 2],
[4, 1]
])
simplices = Delaunay(points).simplices
plt.triplot(points[:, 0], points[:, 1], simplices)
plt.scatter(points[:, 0], points[:, 1], color='r')
plt.show()
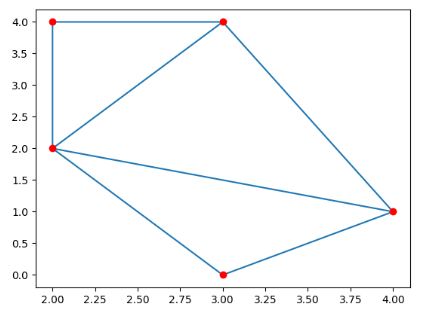
Python Coding Interview Q&A
- Q&A 1
def func(a):
a += 10
return a
def main():
x = 5
y = func(x)
x += y
print(x)
main()
20
- Q&A 2
mydic={"a":1,"b":2,"c":3}
- Q&A 3
my_string = "Python is fun!"
result = my_string[-6:-1] + my_string[-2] + my_string[-8]
print(result)
s funn
- Q&A 4
list_a=[1,2,4,5]
def find_sum_of_the_list(data):
return sum(data)
print(find_sum_of_the_list(list_a))
12
- Q&A 5
def f(x = 100, y = 100):
return(x+y, x-y)
x, y = f(y = 200, x = 100)
print(x, y)
300 -100
- Q&A 6
a=[2,5,3,4]
a[2:2]=[2]
print (a)
[2, 5, 2, 3, 4]
- Q&A 7
print (4/(3*(2-1)))
1.3333333333333333
- Q&A 8
numbers = [1, 2, 3, 4, 5]
squared = [x**2 for x in numbers]
print(squared)
[1, 4, 9, 16, 25]
- Q&A 9
round(0.5)
0
- Q&A 10
24//4//2
3
Conclusions
- Python has emerged as one of the most popular programming languages for developers.
- In this comprehensive guide, we have explored 100 Python projects to learn the basics and beyond.
- These projects will boost your coding skills and provide practical applications across multiple industries and sectors.
- The present guide can help you:
- Build confidence in your ability to create software regardless the level of complexity
- Explore various technologies
- Understand programming concepts better
- Experience a software development life cycle
The Road Ahead
- The next step is to implement the selected 100 data science use-case examples here.
- Enroll in Best Python Courses Online with Certificates.
- Investigate Python for Cloud Computing: Building Scalable Applications with AWS, Azure, and GCP.
Bottom Line:
Accelerate your learning journey across skill levels and domains!
Explore More
- A Complete Python Learn-by-Doing Guide for Aspiring Data Scientists – 1. Basic Programming
- Basic Stock Price Analysis in Python
- Build A Simple NLP/NLTK Chatbot
- A Simple YouTube Download NLP GUI
- A Shamelessly Simple E-Restaurant Order
- Top 6 Reliability/Risk Engineering Learnings
- Effective 2D Image Compression with K-means Clustering
References
- Python Examples
- Python Programs
- Python Tutorial
- Python Program Examples – Simple Code Examples for Beginners
- 25+ Python Programming Examples
- 100 Python projects
- 100+ Python Projects with Source Code
- 100 Python Projects for Practice
- Top 100+ Python Projects for Beginners
- Master Python by building 100 projects in 100 days
- 100 Python Projects for Beginners with solution
- 100+ Machine Learning Projects with Source Code [2024]
- The Big Book of Small Python Projects
- Top Python Projects for Beginners to Advanced [With Source Code]
- 70+ Simple And Advanced Python Projects With Source Code
- 70+ Python Projects for Beginners [Source Code Included]
- 30 Cool, Easy & Fun Python Projects + Source Code [2024]
- 10 basic Python programs for beginners
- Best Python programming examples for beginners and experts
- Practice Python Exercises and Challenges with Solutions
- PyGame: A Primer on Game Programming in Python
- Examples – Practice 50+ Python Programs
- Mastering Pandas: Advanced Techniques for Data Manipulation Excellence
- Ultimate Python Cheat Sheet: Practical Python For Everyday Tasks